C#ICryptoTransform加密判断
时间: 2023-07-12 07:12:45 浏览: 138
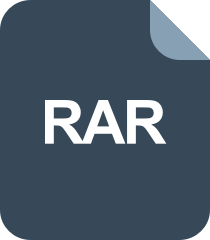
C#加密算法
可以通过以下方法判断C# ICryptoTransform加密是否成功:
1. 使用ICryptoTransform的Transform方法进行加密,将加密后的结果保存到一个byte数组中。
2. 检查byte数组是否为空,如果为空,则加密失败。
3. 检查加密后的byte数组长度是否与原始数据长度相同,如果不同,则加密失败。
4. 使用相同的密钥和算法,对加密后的byte数组进行解密,将解密后的结果保存到一个新的byte数组中。
5. 检查解密后的byte数组是否与原始数据相同,如果相同,则加密成功,否则加密失败。
下面是一个示例代码片段,展示如何使用ICryptoTransform进行加密和解密,并检查加密是否成功:
```
using System;
using System.Security.Cryptography;
class Program
{
static void Main(string[] args)
{
string plaintext = "Hello, world!";
byte[] key = new byte[16];
byte[] iv = new byte[16];
// Create a new instance of the AES algorithm
using (Aes aes = Aes.Create())
{
// Generate a random key and IV
aes.GenerateKey();
aes.GenerateIV();
// Create a new instance of the encryptor
ICryptoTransform encryptor = aes.CreateEncryptor(key, iv);
// Encrypt the plaintext
byte[] ciphertext = encryptor.TransformFinalBlock(
System.Text.Encoding.UTF8.GetBytes(plaintext),
0,
plaintext.Length);
// Check if the ciphertext is null or has different length from plaintext
if (ciphertext == null || ciphertext.Length != plaintext.Length)
{
Console.WriteLine("Encryption failed.");
return;
}
// Create a new instance of the decryptor
ICryptoTransform decryptor = aes.CreateDecryptor(key, iv);
// Decrypt the ciphertext
byte[] decrypted = decryptor.TransformFinalBlock(ciphertext, 0, ciphertext.Length);
// Check if the decrypted plaintext matches the original plaintext
if (System.Text.Encoding.UTF8.GetString(decrypted) == plaintext)
{
Console.WriteLine("Encryption successful.");
}
else
{
Console.WriteLine("Encryption failed.");
}
}
}
}
```
阅读全文
相关推荐

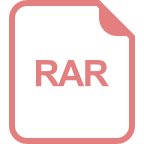
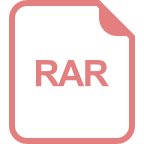
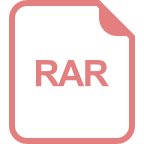
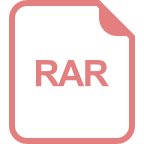
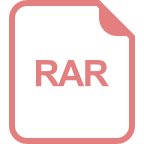
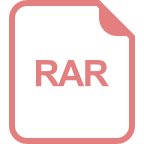
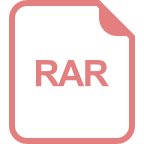
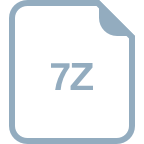
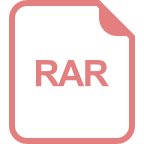
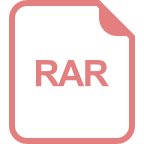
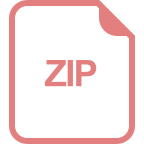
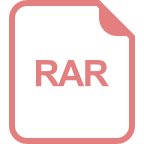
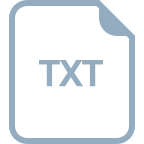
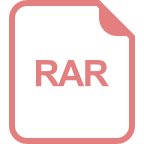
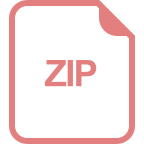