创建一个有6个结点的单向链表,实现结点的增加、删除、查找、移动、遍历显示的功能
时间: 2024-05-15 13:18:23 浏览: 11
好的,我可以帮您解决这个问题。
这里给您提供一个 C++ 的实现示例:
```
#include <iostream>
using namespace std;
// 单向链表结点的定义
struct Node {
int value;
Node* next;
};
// 创建单向链表
Node* createList(int arr[], int n) {
Node* head = new Node; // 创建链表头结点
Node* tail = head;
for (int i = 0; i < n; ++i) {
Node* node = new Node; // 创建新结点
node->value = arr[i];
node->next = nullptr;
tail->next = node; // 将新结点添加到链表尾
tail = node;
}
return head;
}
// 打印单向链表
void printList(Node* head) {
for (Node* p = head->next; p != nullptr; p = p->next) {
cout << p->value << " ";
}
cout << endl;
}
// 在指定位置插入结点
void insertNode(Node* head, int index, int value) {
Node* p = head;
for (int i = 0; i < index && p != nullptr; ++i) {
p = p->next;
}
if (p == nullptr) {
return; // 插入位置越界
}
Node* node = new Node;
node->value = value;
node->next = p->next;
p->next = node;
}
// 删除指定位置的结点
void deleteNode(Node* head, int index) {
Node* p = head;
for (int i = 0; i < index && p->next != nullptr; ++i) {
p = p->next;
}
if (p->next == nullptr) {
return; // 删除位置越界
}
Node* q = p->next;
p->next = q->next;
delete q;
}
// 查找指定值的结点
Node* findNode(Node* head, int value) {
for (Node* p = head->next; p != nullptr; p = p->next) {
if (p->value == value) {
return p;
}
}
return nullptr;
}
// 将指定结点移动到链表头
void moveNode(Node* head, int value) {
Node* p = head;
while (p->next != nullptr && p->next->value != value) {
p = p->next;
}
if (p->next != nullptr) {
Node* q = p->next;
p->next = q->next;
q->next = head->next;
head->next = q;
}
}
int main() {
int arr[6] = {1, 2, 3, 4, 5, 6};
Node* head = createList(arr, 6);
// 打印链表
printList(head);
// 在第3个位置插入结点7
insertNode(head, 3, 7);
printList(head);
// 删除第5个位置的结点
deleteNode(head, 5);
printList(head);
// 查找值为2的结点
Node* node = findNode(head, 2);
if (node != nullptr) {
cout << "Found node: " << node->value << endl;
} else {
cout << "Node not found." << endl;
}
// 移动值为4的结点到链表头
moveNode(head, 4);
printList(head);
return 0;
}
```
希望这个示例代码能够帮助您,如果您还有其他问题,随时欢迎提出。
相关推荐
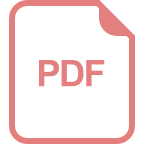
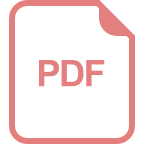
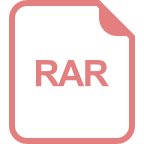










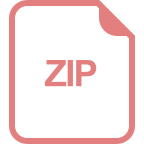
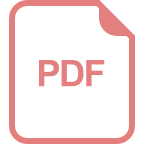
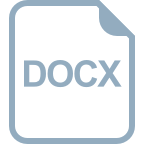
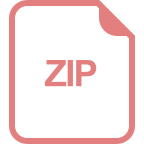
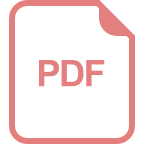