Windows .NET 开发CAN通信
时间: 2023-12-19 13:49:07 浏览: 98
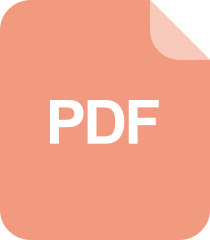
配套开发板实验手册:CAN通信实验.pdf
如果你想在 Windows 上使用 .NET 开发 CAN 通信,可以使用以下步骤:
1. 安装 CAN 适配器的驱动程序:首先,你需要确定你要使用的 CAN 适配器的型号,并从其官方网站上下载和安装驱动程序。
2. 安装 .NET Framework:确保在你的计算机上安装了 .NET Framework。如果没有安装,你可以从 Microsoft 的官方网站上下载并安装它。
3. 安装 CAN 消息库:安装一个能够处理 CAN 消息的 .NET 库,比如 Peak CAN 消息库或 Vector CAN 消息库。这些库提供了一组 .NET 类和方法,可以帮助你发送和接收 CAN 消息。
4. 编写代码:在 Visual Studio 中编写 C# 代码,使用所选的 CAN 消息库的类和方法,实现 CAN 消息的发送和接收。
以下是一个简单的示例代码,使用 Peak CAN 消息库来发送和接收 CAN 消息:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Peak.Can.Light;
namespace CANTest
{
class Program
{
static void Main(string[] args)
{
TPCANHandle h = TPCANHandle.PCAN_USBBUS1;
TPCANMsg msg = new TPCANMsg();
TPCANStatus res;
// Initialize the CAN channel
res = PCANLight.Initialize(h, TPCANBaudrate.PCAN_BAUD_500K);
if (res == TPCANStatus.PCAN_ERROR_OK)
{
Console.WriteLine("CAN channel initialized successfully.");
// Send a CAN message
msg.ID = 0x123;
msg.LEN = 8;
msg.DATA[0] = 0x01;
msg.DATA[1] = 0x02;
msg.DATA[2] = 0x03;
msg.DATA[3] = 0x04;
msg.DATA[4] = 0x05;
msg.DATA[5] = 0x06;
msg.DATA[6] = 0x07;
msg.DATA[7] = 0x08;
res = PCANLight.Write(h, ref msg);
if (res == TPCANStatus.PCAN_ERROR_OK)
{
Console.WriteLine("CAN message sent successfully.");
// Receive a CAN message
TPCANMsg recvMsg = new TPCANMsg();
res = PCANLight.Read(h, out recvMsg, null);
if (res == TPCANStatus.PCAN_ERROR_OK)
{
Console.WriteLine("CAN message received: ID={0:X}, LEN={1}, DATA={2}", recvMsg.ID, recvMsg.LEN, BitConverter.ToString(recvMsg.DATA));
}
else
{
Console.WriteLine("Error receiving CAN message: {0}", res);
}
}
else
{
Console.WriteLine("Error sending CAN message: {0}", res);
}
// Close the CAN channel
PCANLight.Uninitialize(h);
}
else
{
Console.WriteLine("Error initializing CAN channel: {0}", res);
}
Console.ReadLine();
}
}
}
```
这段代码使用 Peak CAN 消息库来初始化 CAN 通道,发送一个 CAN 消息并接收一个 CAN 消息。你需要根据所选的 CAN 消息库修改代码。
阅读全文
相关推荐
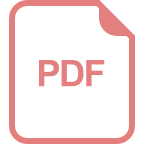
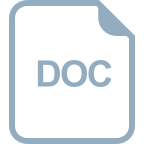
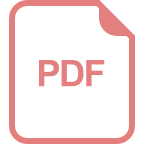
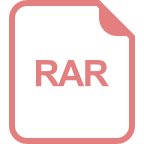
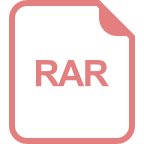
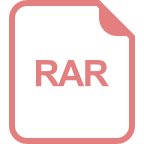
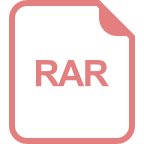
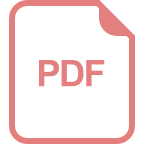
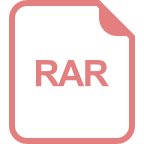
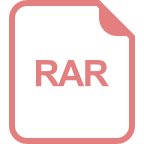
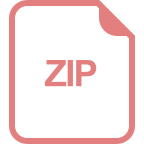
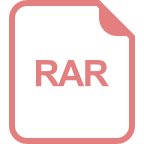
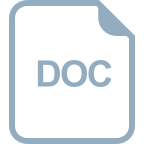
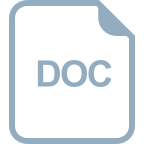
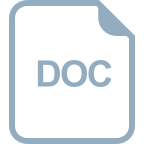
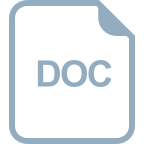