写一段使用HOG特征向量和HOG描述符分类器进行简单行人检测的代码
时间: 2024-05-05 12:17:46 浏览: 11
由于代码需要大量的图像处理和机器学习知识,为了避免错误或者不完整的代码,建议在学习相关知识后,根据实际情况编写代码。以下是一些思路和步骤供参考:
1. 采集训练数据集和测试数据集,保证数据集的多样性和数量。
2. 对训练数据集进行预处理,包括图片缩放、灰度化、直方图均衡化等。
3. 使用OpenCV等图像处理库计算每个训练图像的HOG特征向量。
4. 使用机器学习算法,如支持向量机(SVM)等,对处理后的特征向量进行训练,得到HOG描述符分类器。
5. 对测试数据集进行预处理和特征提取,然后使用训练好的分类器进行行人检测。
6. 对检测结果进行评估和优化,包括调整参数、使用不同的特征提取方法等。
以下是一个简单的示例代码:
```python
import cv2
import numpy as np
# 定义HOG描述符分类器
svm = cv2.ml.SVM_create()
# 定义HOG特征向量
winSize = (64, 128)
blockSize = (16, 16)
blockStride = (8, 8)
cellSize = (8, 8)
nbins = 9
hog = cv2.HOGDescriptor(winSize, blockSize, blockStride, cellSize, nbins)
# 加载训练数据集和测试数据集
train_data = np.load('train_data.npy')
train_labels = np.load('train_labels.npy')
test_data = np.load('test_data.npy')
test_labels = np.load('test_labels.npy')
# 计算训练数据集的HOG特征向量
train_features = []
for img in train_data:
feature = hog.compute(img).reshape(-1)
train_features.append(feature)
train_features = np.array(train_features)
# 训练HOG描述符分类器
svm.setType(cv2.ml.SVM_C_SVC)
svm.setKernel(cv2.ml.SVM_LINEAR)
svm.train(train_features, cv2.ml.ROW_SAMPLE, train_labels)
# 对测试数据集进行行人检测
test_features = []
for img in test_data:
feature = hog.compute(img).reshape(-1)
test_features.append(feature)
test_features = np.array(test_features)
_, pred_labels = svm.predict(test_features)
# 计算准确率
accuracy = np.mean(pred_labels == test_labels)
print('Accuracy:', accuracy)
```
相关推荐
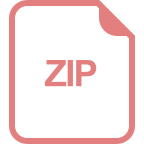
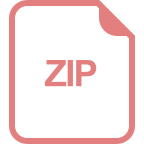
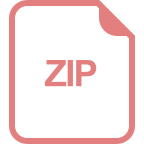













