matlab电动汽车路径优化的遗传算法求解代码
时间: 2023-08-23 09:04:40 浏览: 51
以下是一个简单的 MATLAB 代码示例,用遗传算法优化电动汽车路径:
```matlab
function [bestRoute, bestFitness] = gaEVPathOptimization()
% 参数设置
numCities = 20; % 城市数量
numGenerations = 1000; % 迭代次数
popSize = 100; % 种群数量
eliteRatio = 0.1; % 精英比例
mutationRate = 0.02; % 变异率
% 随机生成城市坐标
cities = 10 * rand(numCities, 2);
% 初始化种群
population = zeros(popSize, numCities);
for i = 1:popSize
population(i,:) = randperm(numCities);
end
% 进化循环
for i = 1:numGenerations
% 计算适应度
fitness = zeros(popSize, 1);
for j = 1:popSize
fitness(j) = evaluateFitness(population(j,:), cities);
end
% 找出精英个体
eliteNum = round(eliteRatio * popSize);
[sortedFitness, sortedIdx] = sort(fitness, 'descend');
elitePopulation = population(sortedIdx(1:eliteNum),:);
% 选择
parent1 = elitePopulation(randi(eliteNum), :);
parent2 = elitePopulation(randi(eliteNum), :);
child = crossover(parent1, parent2);
% 变异
if rand < mutationRate
child = mutate(child);
end
% 替换
idx = randi(popSize);
population(idx,:) = child;
end
% 计算最佳路径和适应度
bestFitness = Inf;
for i = 1:popSize
f = evaluateFitness(population(i,:), cities);
if f < bestFitness
bestFitness = f;
bestRoute = population(i,:);
end
end
% 绘制最佳路径
figure;
plot(cities(:,1), cities(:,2), 'o');
hold on;
plot(cities([bestRoute, bestRoute(1)], 1), cities([bestRoute, bestRoute(1)], 2), '-r');
title(sprintf('Total distance: %0.2f', bestFitness));
end
function fitness = evaluateFitness(route, cities)
% 计算路径距离
distance = 0;
for i = 1:length(route)-1
distance = distance + norm(cities(route(i),:) - cities(route(i+1),:));
end
distance = distance + norm(cities(route(end),:) - cities(route(1),:));
% 计算适应度
fitness = 1 / distance;
end
function child = crossover(parent1, parent2)
% 交叉操作:部分映射交叉
n = length(parent1);
child = zeros(1, n);
start = randi(n);
stop = randi(n);
if start > stop
temp = start;
start = stop;
stop = temp;
end
child(start:stop) = parent1(start:stop);
idx = 1;
for i = 1:n
if ~ismember(parent2(i), child)
while child(idx) ~= 0
idx = idx + 1;
end
child(idx) = parent2(i);
end
end
end
function child = mutate(parent)
% 变异操作:随机交换两个基因
n = length(parent);
idx1 = randi(n);
idx2 = randi(n);
temp = parent(idx1);
parent(idx1) = parent(idx2);
parent(idx2) = temp;
child = parent;
end
```
这个示例代码使用遗传算法来优化电动汽车经过一系列城市的路径。在代码中,我们首先随机生成了一些城市坐标,并将它们表示为一个 $n \times 2$ 的矩阵。然后,我们初始化了一个由 $popSize$ 个个体组成的种群,每个个体表示一个遍历所有城市的路径。在进化循环中,我们计算每个个体的适应度(路径总长度的倒数),并选择一些精英个体进行繁殖。每次繁殖操作都会选择两个精英个体,进行交叉和变异操作,然后将产生的子代替换掉种群中的某个个体。经过多次迭代后,我们可以找到最佳路径和最短距离,并绘制出来。
相关推荐
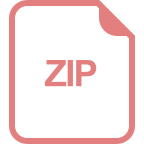
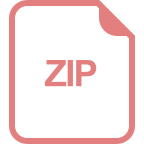














