用vue3写雪花算法
时间: 2023-08-26 22:11:59 浏览: 74
### 回答1:
好的,以下是使用Vue 3编写雪花算法的示例代码:
在template模板中,我们可以使用canvas元素来绘制雪花,canvas可以用来绘制各种形状、图形和动画,这里我们用canvas来绘制雪花的形状。
```
<template>
<div>
<canvas ref="canvas" :width="canvasWidth" :height="canvasHeight"></canvas>
</div>
</template>
```
在script脚本中,我们可以在mounted钩子中获取canvas元素,然后获取canvas的上下文对象,通过绘制函数drawFlake绘制雪花。
```
<script>
export default {
data() {
return {
canvasWidth: 600,
canvasHeight: 600,
flakeCount: 400,
flakes: [],
}
},
mounted() {
this.canvas = this.$refs.canvas
this.ctx = this.canvas.getContext('2d')
this.drawFlake()
},
methods: {
drawFlake() {
for (let i = 0; i < this.flakeCount; i++) {
const x = Math.floor(Math.random() * this.canvasWidth)
const y = Math.floor(Math.random() * this.canvasHeight)
const radius = Math.floor(Math.random() * 5) + 2
const opacity = Math.random()
this.flakes.push({ x, y, radius, opacity })
}
requestAnimationFrame(this.drawFlake)
this.updateFlakes()
},
updateFlakes() {
this.ctx.clearRect(0, 0, this.canvasWidth, this.canvasHeight)
for (let flake of this.flakes) {
this.ctx.beginPath()
this.ctx.arc(flake.x, flake.y, flake.radius, 0, 2 * Math.PI)
this.ctx.fillStyle = `rgba(255, 255, 255, ${flake.opacity})`
this.ctx.fill()
flake.y += 1
if (flake.y > this.canvasHeight) {
flake.y = 0
}
}
requestAnimationFrame(this.updateFlakes)
},
},
}
</script>
```
在以上代码中,我们使用了data函数来定义数据变量canvasWidth、canvasHeight、flakeCount和flakes,其中canvasWidth和canvasHeight定义了canvas的宽度和高度,flakeCount定义了雪花的数量,flakes数组用来存储雪花的数据。
在mounted钩子中,我们获取了canvas元素和canvas的上下文对象ctx,并且调用了drawFlake函数,在drawFlake函数中,我们使用for循环来生成雪花的数据,包括x、y坐标、半径和透明度,并且将雪花的数据保存在flakes数组中。在每次绘制雪花之前,我们使用requestAnimationFrame方法来请求动画帧,然后调用updateFlakes函数。
在updateFlakes函数中,我们首先清除了canvas上的绘制,然后使用for循环绘制每一个雪花,并且设置雪花的颜色、位置和透明度,然后让雪花向下移动,并且判断雪花是否
### 回答2:
雪花算法是一种分布式ID生成算法,主要用于保证在分布式环境下生成的ID的全局唯一性。Vue 3是一种用于构建用户界面的JavaScript框架。如果要使用Vue 3编写雪花算法,需要遵循以下几个步骤:
1. 导入vue和相关依赖:在Vue 3项目中,首先需要导入Vue和其他需要使用的依赖库,可以使用`import`语句来导入。
2. 实现雪花算法:雪花算法的实现主要包括生成ID的方法。在Vue 3中,可以将雪花算法的代码封装在组件中,使用`methods`选项定义生成ID的方法。
3. 在Vue组件中使用雪花算法:在Vue组件中,可以使用生成的ID作为标识符,通过调用雪花算法的生成ID方法来获取ID值。可以在`data`选项中定义一个变量来存储生成的ID值,并且在需要使用ID的地方引用该变量。
4. 绑定ID到用户界面:将生成的ID值绑定到用户界面中,可以使用Vue 3的指令来实现。例如,可以使用`v-bind`指令将生成的ID绑定到HTML元素的属性中,或者使用`{{}}`插值语法将ID值显示在界面上。
5. 运行Vue应用:最后,使用Vue 3提供的命令来运行Vue应用,将生成的ID在用户界面中展示出来。
使用Vue 3编写雪花算法的关键在于在组件中封装算法并运用到用户界面中。通过以上步骤,可以实现基于Vue 3的雪花算法。
### 回答3:
雪花算法是一种生成唯一ID的算法,可以在分布式系统中避免ID的重复。下面是使用Vue3编写一个简单的雪花算法的示例代码:
```javascript
// 雪花算法的实现代码
class SnowFlake {
constructor(dataCenterId, workerId) {
this.dataCenterId = dataCenterId;
this.workerId = workerId;
this.sequence = 0;
this.lastTimestamp = -1;
}
generateId() {
let currentTimestamp = this.getCurrentTimestamp();
if (this.lastTimestamp === currentTimestamp) {
this.sequence = (this.sequence + 1) & 4095;
if (this.sequence === 0) {
currentTimestamp = this.getNextTimestamp();
}
} else {
this.sequence = 0;
}
this.lastTimestamp = currentTimestamp;
return (
(currentTimestamp - 1610649600000) << 22 | // 时间戳占用的位数为41位,选择从2021-01-15 00:00:00开始计算
(this.dataCenterId << 17) |
(this.workerId << 12) |
this.sequence
);
}
getCurrentTimestamp() {
return new Date().getTime();
}
getNextTimestamp() {
let timestamp = this.getCurrentTimestamp();
while (timestamp <= this.lastTimestamp) {
timestamp = this.getCurrentTimestamp();
}
return timestamp;
}
}
// 在Vue3中使用雪花算法的示例代码
const { ref } = Vue;
const App = {
setup() {
const dataCenterId = ref(0);
const workerId = ref(0);
const id = ref('');
const generateId = () => {
const snowFlake = new SnowFlake(dataCenterId.value, workerId.value);
id.value = snowFlake.generateId().toString();
};
return {
dataCenterId,
workerId,
id,
generateId
};
},
template: `
<div>
<label for="dataCenterId">Data Center ID:</label>
<input id="dataCenterId" type="number" v-model="dataCenterId" />
<br />
<label for="workerId">Worker ID:</label>
<input id="workerId" type="number" v-model="workerId" />
<br />
<button @click="generateId">Generate ID</button>
<br />
<div v-if="id !== ''">ID: {{ id }}</div>
</div>
`
};
Vue.createApp(App).mount('#app');
```
在上面的示例代码中,我们创建了一个SnowFlake类,其中包含了生成唯一ID的逻辑。在Vue3的应用中,我们使用`ref`来定义了数据中心ID和工作者ID的响应式变量,并在`generateId`方法中使用这些变量创建雪花对象并生成唯一ID。在模板中,我们通过绑定输入框和按钮的点击事件来获取用户输入的数据,并显示生成的唯一ID。
相关推荐
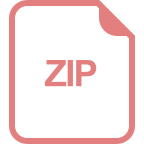
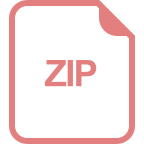
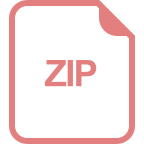
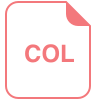
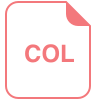
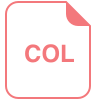
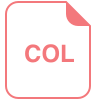
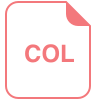









