#include <windows.h> #include <iostream> using namespace std; void play_heap(HANDLE heap) { char* p = (char*)HeapAlloc(heap, 0, 100); int i; for (i = 0; i < 10; i++) { *(p + i) = 'A' + i; } *(p+i) = '\0'; cout << p << endl; HeapFree(heap, 0, p); } int main() { HANDLE default_heap = GetProcessHeap(); play_heap(default_heap); HANDLE new_heap = HeapCreate(HEAP_NO_SERIALIZE, 1000, 2000); play_heap(new_heap); HeapDestroy(new_heap); return 0; }
时间: 2024-01-03 19:04:07 浏览: 34
这段代码是一个简单的使用 Windows API 的堆内存操作示例。具体来说,它使用了以下几个函数:
- `GetProcessHeap`:获取进程的默认堆句柄。
- `HeapCreate`:创建一个新的堆并返回其句柄。
- `HeapAlloc`:从堆中分配一块指定大小的内存,并返回指向该内存块的指针。
- `HeapFree`:释放之前分配的堆内存。
- `HeapDestroy`:销毁之前创建的堆并释放其所有内存。
在 `main` 函数中,首先获取了进程的默认堆句柄 `default_heap`,并调用了 `play_heap` 函数,对其进行了一次内存分配和释放操作。`play_heap` 函数中,先使用 `HeapAlloc` 分配了一块 100 字节的内存,并将其中前 10 个字节写入了字母 A 到 J,最后加上了一个字符串结束符。然后输出该内存块的内容,并使用 `HeapFree` 将其释放。
接下来,使用 `HeapCreate` 创建了一个新的堆 `new_heap`,并重新调用了 `play_heap` 函数,对其进行了一次类似的内存分配和释放操作。最后使用 `HeapDestroy` 销毁了该堆并释放了其中所有的内存。
需要注意的是,代码中对堆的操作是没有加锁的(使用了 `HEAP_NO_SERIALIZE` 标志),因此在多线程环境下可能会发生竞争条件。如果需要在多线程中使用堆,应该使用 `HEAP_GENERATE_EXCEPTIONS` 或 `HEAP_CREATE_ENABLE_EXECUTE` 标志来确保线程安全。
相关问题
#include<iostream> #include<stdlib.h> #include<time.h> #include<windows.h> #define+M+100+ using+name
这段代码是C++代码,它包含了一些头文件和宏定义,并定义了一个main函数。在main函数中,它使用了一个while循环来读取输入的半径值,并计算圆的面积,然后输出结果。代码中使用了cmath库中的pow函数来计算半径的平方,使用了iomanip库中的setprecision函数来设置输出结果的精度。
这段代码的功能是计算输入半径的圆的面积,并输出结果。
以下是这段代码的解释和演示:
```cpp
#include<iostream>
#include<stdlib.h>
#include<time.h>
#include<windows.h>
#define M 100
using namespace std;
int main(void) {
int r;
while ( cin >> r && r <= 10000 && r >= 1 && r != EOF ) {
double s;
s = PI * pow(r,2);
cout << fixed << setprecision(7) << s << endl;
}
return 0;
}
```
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <windows.h> #include <malloc.h>改成c++
在C++中,`<stdio.h>`、`<stdlib.h>`、`<string.h>`和`<windows.h>`这些头文件在C++标准库中对应的是`iostream`、`cstdlib`、`string`以及`Windows.h`(注意,C++不支持`windows.h`,因为它主要用于Windows API)。为了将这段C代码转换为C++,我们需要做以下调整:
1. 使用`#include <iostream>`替换`#include <stdio.h>`,因为`iostream`包含了输入输出流和基本I/O操作。
2. 使用`#include <cstdlib>`替换`#include <stdlib.h>`,因为`cstdlib`包含了内存管理函数。
3. 使用`#include <string>`替换`#include <string.h>`,因为`string`库提供了字符串处理功能。
4. 删除`#include <windows.h>`,因为C++不支持Windows API,如果需要Windows特定的头文件,需要使用C++的命名空间或头文件,但这里假设你不需要Windows API。
下面是转换后的C++代码:
```cpp
#include <iostream>
#include <cstdlib>
#include <string>
// 使用std::string代替char数组
std::string strchr(const std::string& s, char c) {
auto it = std::find(s.begin(), s.end(), c);
if (it != s.end()) {
return std::string{s.begin(), it};
}
return "";
}
// 使用std::string和strcpy的等价函数std::copy
std::string mystr(std::string& dst, const std::string& str) {
std::copy(str.begin(), str.end(), dst.begin());
dst.resize(dst.size()); // 添加终止符'\0'
return dst;
}
int main() {
std::string s1 = "abc";
std::string s2 = mystr(s1);
std::cout << s2 << std::endl;
return 0;
}
```
相关推荐
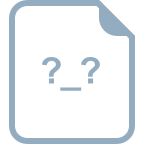














