datagridview绑定数据源,每行添加按钮,用代码实现
时间: 2023-09-03 17:48:11 浏览: 132
### 回答1:
可以通过以下步骤实现在DataGridView中添加按钮并绑定事件:
1. 在DataGridView中添加一个DataGridViewButtonColumn列,用来放置按钮。
```csharp
DataGridViewButtonColumn buttonColumn = new DataGridViewButtonColumn();
buttonColumn.HeaderText = "操作";
buttonColumn.Text = "删除";
buttonColumn.UseColumnTextForButtonValue = true;
dataGridView1.Columns.Add(buttonColumn);
```
2. 在DataGridView的CellClick事件中添加按钮点击事件处理程序。
```csharp
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == dataGridView1.Columns["操作"].Index)
{
//处理按钮点击事件
MessageBox.Show("您点击了第 " + e.RowIndex + " 行的删除按钮");
}
}
```
3. 将数据源绑定到DataGridView。
```csharp
DataTable dt = new DataTable();
dt.Columns.Add("编号");
dt.Columns.Add("姓名");
dt.Rows.Add("001", "张三");
dt.Rows.Add("002", "李四");
dataGridView1.DataSource = dt;
```
完整的代码示例:
```csharp
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
InitDataGridView();
}
private void InitDataGridView()
{
//添加按钮列
DataGridViewButtonColumn buttonColumn = new DataGridViewButtonColumn();
buttonColumn.HeaderText = "操作";
buttonColumn.Text = "删除";
buttonColumn.UseColumnTextForButtonValue = true;
dataGridView1.Columns.Add(buttonColumn);
//绑定数据源
DataTable dt = new DataTable();
dt.Columns.Add("编号");
dt.Columns.Add("姓名");
dt.Rows.Add("001", "张三");
dt.Rows.Add("002", "李四");
dataGridView1.DataSource = dt;
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == dataGridView1.Columns["操作"].Index)
{
//处理按钮点击事件
MessageBox.Show("您点击了第 " + e.RowIndex + " 行的删除按钮");
}
}
}
```
### 回答2:
在DataGridView中为每行添加按钮,可以通过DataGridView的CellFormatting事件来实现。具体步骤如下:
1. 首先,确保已经将数据源绑定到DataGridView上,可以通过设置DataGridView的DataSource属性来实现。
2. 在DataGridView的CellFormatting事件中添加代码,该事件会在每个单元格绘制时触发。
3. 在CellFormatting事件中,首先判断当前单元格所在列是否为按钮列,可以通过列的索引或者名称进行判断。例如,若按钮列是第一列,可以使用以下代码判断:
```
if (e.ColumnIndex == 0)
{
// 添加按钮代码
}
```
4. 在判断当前单元格所在列为按钮列后,判断当前单元格所在行是否是数据行,而非标题行或者其他特殊行。可以通过判断单元格所在行的索引进行判断。
```
if (e.RowIndex >= 0)
{
// 添加按钮代码
}
```
5. 在判断为数据行后,创建一个按钮控件,并将其添加到当前单元格的控件集合中。
```
if (e.RowIndex >= 0)
{
DataGridViewButtonCell buttonCell = new DataGridViewButtonCell();
dataGridView.Rows[e.RowIndex].Cells[0] = buttonCell;
}
```
6. 此时,按钮已经添加到了DataGridView的每一行上。
完整代码示例如下所示:
```
private void dataGridView_CellFormatting(object sender, DataGridViewCellFormattingEventArgs e)
{
if (e.ColumnIndex == 0 && e.RowIndex >= 0)
{
DataGridViewButtonCell buttonCell = new DataGridViewButtonCell();
dataGridView.Rows[e.RowIndex].Cells[0] = buttonCell;
}
}
```
注意:该示例假设按钮列为第一列,若按钮列的索引不是0时,需要根据实际情况进行修改。另外,可以根据需求对按钮样式、事件等进行定制。
阅读全文
相关推荐
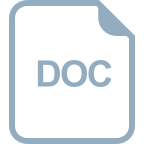
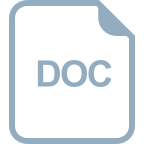
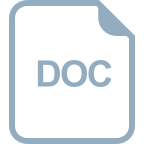

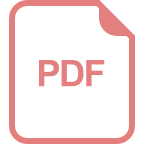
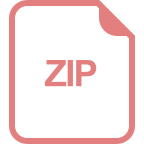
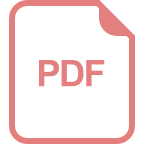
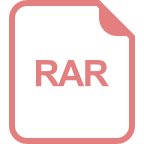
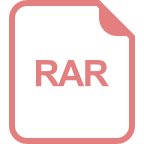
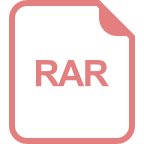
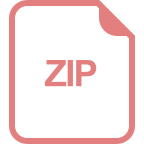
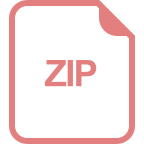
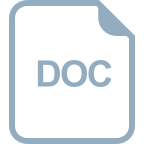
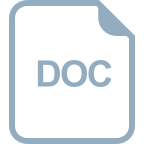
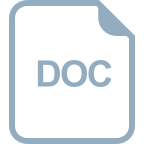



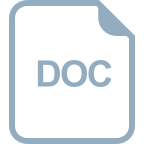