Vue使用SVG制作一个横向树形结构
时间: 2024-02-23 14:02:38 浏览: 86
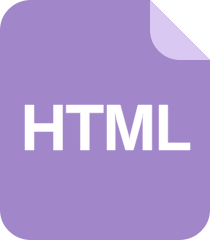
VUE 实现树形结构
要使用SVG制作一个横向树形结构,可以按以下步骤进行:
1. 首先,使用Vue创建一个组件,命名为Tree。
2. 在Tree组件中,使用SVG标签来绘制树形结构。SVG是一种可缩放矢量图形格式,可以通过代码来描述图形,非常适合用来绘制图形化的数据结构。
3. 在SVG标签中,使用<g>标签来组合多个图形元素,形成一个组合。
4. 使用<path>标签来绘制树形结构中的线条。可以使用<path>标签的d属性来定义绘制路径,例如M x1 y1 L x2 y2表示从点(x1,y1)到点(x2,y2)绘制一条直线。
5. 使用<circle>标签来绘制树形结构中的节点。可以使用<circle>标签的cx和cy属性来定义圆心坐标,r属性来定义半径。
6. 在Tree组件中,使用props属性来接收传入的树形结构数据。可以使用递归的方式,将树形结构数据中的每个节点都绘制出来。
下面是一个简单的示例代码:
```html
<template>
<svg>
<g>
<path :d="line.path" stroke="black" fill="none" />
<circle :cx="node.x" :cy="node.y" :r="node.r" fill="red" />
<text :x="node.x" :y="node.y">{{ node.value }}</text>
</g>
<tree v-for="child in node.children" :node="child" :key="child.id" />
</svg>
</template>
<script>
export default {
name: "Tree",
props: {
node: {
type: Object,
required: true,
},
},
computed: {
line() {
const { x, y, width, height } = this.node;
const path = `M ${x} ${y + height / 2} H ${x + width}`;
return { path };
},
},
components: {
Tree,
},
};
</script>
```
在这个示例中,我们定义了一个Tree组件,接收一个node属性作为参数。每个Tree组件都会绘制一个节点和一条线条,然后递归地绘制子节点。在计算属性line中,我们使用节点的位置和尺寸信息来计算线条的路径。在模板中,我们使用<g>标签来组合多个图形元素,使用v-for指令来遍历子节点,并递归地渲染Tree组件。
当我们使用这个Tree组件时,可以将树形结构数据作为参数传入:
```html
<template>
<tree :node="treeData" />
</template>
<script>
import Tree from "./Tree.vue";
export default {
name: "App",
components: {
Tree,
},
data() {
return {
treeData: {
id: 1,
value: "root",
children: [
{
id: 2,
value: "child 1",
children: [
{
id: 4,
value: "grandchild 1",
children: [],
},
{
id: 5,
value: "grandchild 2",
children: [],
},
],
},
{
id: 3,
value: "child 2",
children: [],
},
],
},
};
},
};
</script>
```
这样就可以在Vue中使用SVG绘制出一个横向树形结构了。
阅读全文
相关推荐
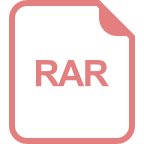
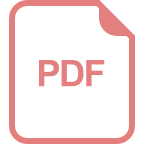
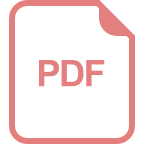
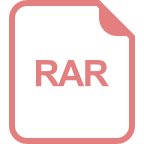
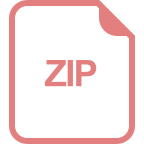
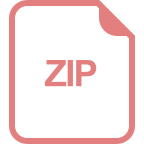
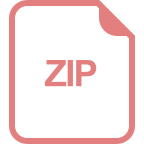
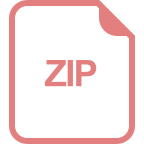
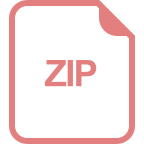
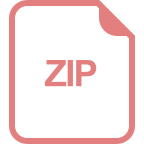
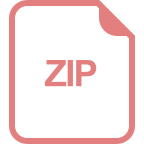
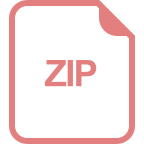
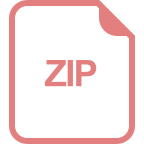
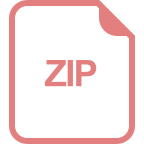
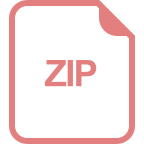
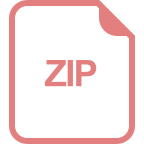
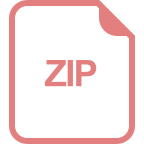