利用pyqt6做一个简易计算器
时间: 2024-05-12 12:02:26 浏览: 172
好的,以下是一个简单的基于 PyQt6 的计算器程序:
```python
from PyQt6.QtWidgets import QApplication, QWidget, QVBoxLayout, QHBoxLayout, QPushButton, QLineEdit
import sys
class Calculator(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("PyQt6 Calculator")
self.setGeometry(100, 100, 300, 300)
self.layout = QVBoxLayout()
self.textbox = QLineEdit()
self.textbox.setFixedHeight(40)
self.layout.addWidget(self.textbox)
buttons_layout = QHBoxLayout()
buttons = [
"7", "8", "9", "/",
"4", "5", "6", "*",
"1", "2", "3", "-",
"0", ".", "C", "+",
"="
]
for button in buttons:
button_obj = QPushButton(button)
button_obj.setFixedSize(50, 50)
buttons_layout.addWidget(button_obj)
button_obj.clicked.connect(self.button_pressed)
self.layout.addLayout(buttons_layout)
self.setLayout(self.layout)
def button_pressed(self):
button = self.sender()
if button.text() == "=":
try:
result = str(eval(self.textbox.text()))
self.textbox.setText(result)
except:
self.textbox.setText("Error")
elif button.text() == "C":
self.textbox.setText("")
else:
self.textbox.setText(self.textbox.text() + button.text())
if __name__ == "__main__":
app = QApplication(sys.argv)
calculator = Calculator()
calculator.show()
sys.exit(app.exec())
```
该程序使用 PyQt6 创建了一个简单的计算器,用户可以通过按下按钮进行数字和操作符输入,并在文本框中查看结果。程序通过 `eval()` 函数计算文本框中的表达式,并在文本框中显示结果。
阅读全文
相关推荐
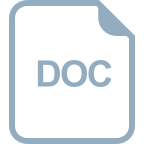
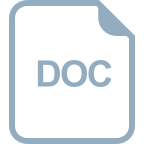
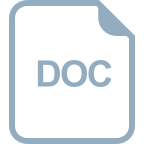



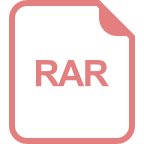
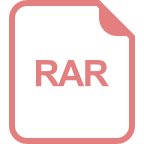
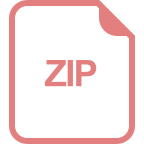
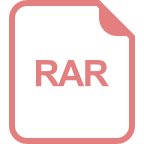
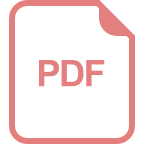
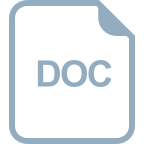
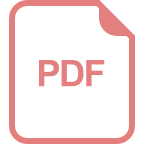
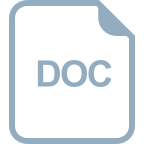
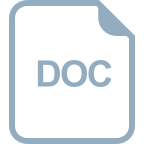
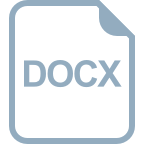
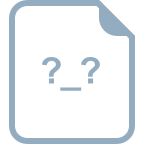