如何用C#把本地文件夹的图片迁移到共享文件夹里?完成后删除本地文件夹里的图片
时间: 2024-10-17 15:12:31 浏览: 27
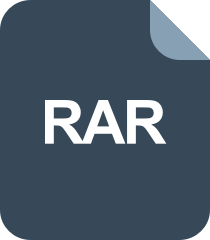
C#Onnx模型信息查看工具 可执行程序exe包

在C#中,你可以使用System.IO命名空间下的Directory类和File类来操作文件和目录。以下是将本地图片迁移至共享文件夹并删除本地文件的操作步骤:
1. 首先,需要获取本地图片的路径以及目标共享文件夹的路径。假设本地图片路径为`localImagePath`,共享文件夹路径为`sharedFolderPath`。
```csharp
string localImagePath = "C:\\Local\\Image.jpg";
string sharedFolderPath = "\\Server\\SharedFolder"; // 根据实际情况填写网络路径
```
2. 使用`Directory.CreateDirectory()`检查共享文件夹是否存在,如果不存在则创建它。
```csharp
if (!Directory.Exists(sharedFolderPath))
{
Directory.CreateDirectory(sharedFolderPath);
}
```
3. 然后,使用`File.Copy()`方法复制图片到共享文件夹。
```csharp
try
{
File.Copy(localImagePath, sharedFolderPath + Path.GetFileName(localImagePath), true); // 第三个参数true表示覆盖同名文件
}
catch (Exception ex)
{
Console.WriteLine($"Error copying file: {ex.Message}");
}
```
4. 最后,删除本地图片。同样使用`File.Delete()`方法,记得添加异常处理以防止错误发生。
```csharp
try
{
File.Delete(localImagePath);
Console.WriteLine("Local image deleted successfully.");
}
catch (Exception ex)
{
Console.WriteLine($"Error deleting local file: {ex.Message}");
}
```
完整的示例代码:
```csharp
using System;
using System.IO;
class Program
{
static void Main(string[] args)
{
string localImagePath = "C:\\Local\\Image.jpg";
string sharedFolderPath = "\\Server\\SharedFolder";
if (!Directory.Exists(sharedFolderPath))
Directory.CreateDirectory(sharedFolderPath);
try
{
File.Copy(localImagePath, sharedFolderPath + Path.GetFileName(localImagePath), true);
Console.WriteLine("Moving image to shared folder...");
File.Delete(localImagePath);
Console.WriteLine("Local image deleted.");
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
```
阅读全文
相关推荐
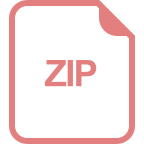
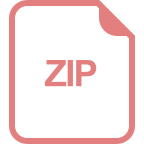

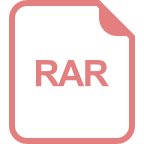
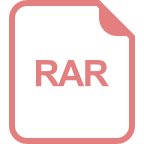
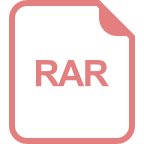
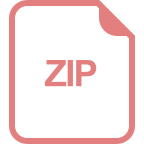
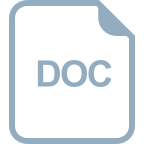
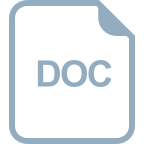
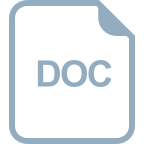
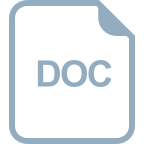
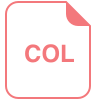
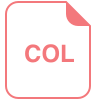
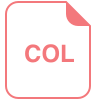
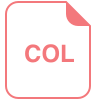
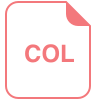