vue3 setup怎么写created
时间: 2023-11-17 21:06:38 浏览: 170
在 Vue 3 中,使用 `setup()` 函数来替代 Vue 2.x 中的 `created()` 钩子函数。 `setup()` 函数会在组件实例创建之前执行,并且可以访问到组件的 props、data、computed、methods 等。
你可以在 `setup()` 函数中返回一个对象,该对象的属性将会被暴露给模板。如果你想要执行一些操作,可以在 `setup()` 中使用普通的 JavaScript 代码。
下面是一个使用 `setup()` 函数并添加类似 `created()` 钩子函数的示例:
```
<template>
<div>{{ message }}</div>
</template>
<script>
import { ref } from 'vue'
export default {
setup() {
const message = ref('Hello, world!')
// 类似 created 钩子函数
const created = () => {
console.log('created')
}
created()
return { message }
}
}
</script>
```
在上面的代码中,我们使用了 `ref()` 函数来创建一个响应式的数据。然后我们定义了一个 `created()` 函数,使用它来在组件实例创建后执行一些操作。最后,我们将 `message` 数据暴露给模板。
相关问题
vue3setup中如何使用created
为了在Vue 3的setup()函数中使用created()生命周期钩子函数,我们需要将其转换为一个Ref对象,并在setup()函数中返回一个对象来访问它。具体的代码如下所示:
```javascript
import { onCreated, ref } from 'vue';
export default {
setup() {
const myData = ref(null);
onCreated(() => {
myData.value = 'Hello, world!';
});
return { myData };
},
};
```
在这个例子中,我们使用了Vue 3的onCreated()函数来模拟created()生命周期钩子函数。我们也使用了一个Ref对象来存储我们的数据。最后,我们在setup()函数中返回一个对象,用于从组件外部访问我们的数据。
请注意,如果您使用的是Vue 3 Composition API而不是Options API,那么您不应该使用created()生命周期钩子函数。相反,您应该使用onMounted()钩子函数来代替它,并在其中执行初始化代码。
vue3 setup
Vue 3 introduces a new function called `setup` to replace the options-based API used in Vue 2. The `setup` function allows you to initialize component state, define computed properties, and set up component lifecycle hooks.
In Vue 3, the `setup` function is executed before the component is created, providing a more explicit and flexible way to define component logic. Here's an example of how to use the `setup` function:
```javascript
<template>
<div>
<p>{{ message }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const message = ref('Hello Vue 3');
function increment() {
message.value += '!';
}
return {
message,
increment
};
}
};
</script>
```
In the example above, we import `ref` from the `vue` package, which allows us to create a reactive reference. Inside the `setup` function, we declare a `message` variable using `ref`, and a function called `increment` that appends an exclamation mark to the message. We then return these variables and functions as an object from the `setup` function.
By using the `setup` function, you can access reactive properties and functions directly in the template without the need for the `this` context. This makes the code more concise and easier to read.
I hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
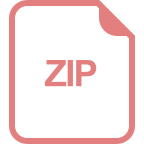
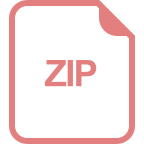













