string *p = new string[n];
时间: 2024-06-06 11:06:25 浏览: 2
This code dynamically allocates an array of n strings and returns a pointer to the first element of the array.
The asterisk (*) in front of p indicates that p is a pointer variable. The data type of the pointer variable is string, which means that it can store the memory address of a string object.
The keyword "new" is used to allocate memory dynamically at runtime. In this case, it allocates memory for an array of n strings on the heap.
Overall, this line of code creates an array of n strings that can be accessed using pointer p.
相关问题
int main() { int** way = NULL; float* D = NULL; int s=0, e=0, p=0; int n = 1; int* c; c = new int[10]; string start, end; //读取 city 表 City* cities = NULL; Cities(cities); //读取 route 表 Route* route = NULL; Routes(route); //建立邻接矩阵 Graph graph; CreatGraph(graph, cities, route);
这段代码是用 C++ 实现的,主要包括以下内容:
1. 定义了一个指向指针的指针 way,和一个指向 float 类型的指针 D,以及 s、e、p 三个整型变量和一个整型变量 n。
2. 动态分配了一个长度为 10 的整型数组 c。
3. 定义了两个字符串类型的变量 start 和 end。
4. 调用 Cities 函数和 Routes 函数分别从 city 表和 route 表中读取数据,并将数据存储在 cities 和 route 指针变量中。
5. 调用 CreatGraph 函数建立邻接矩阵,该函数接受三个参数,第一个参数是一个 Graph 类型的变量,第二个参数是一个 City 类型的指针,第三个参数是一个 Route 类型的指针。
以下这段代码有什么问题吗: #include <stdio.h> /* 在get1() 中,定义的buf属于占空间,当函数结束后,buf 的内存空间已经被释放,而返回的指针仍然指向这块已经释放的内存空间,在使用这个指针时可能会导致程序崩溃或产生未定义行为。所以main函数中 m 为一个野指针*/ void *get1(){ char buf[12] = {"abc"}; return buf; } void get2(int *p){ p = new int[10]; } int main() { int *m; get2(m); //属于值传递,应为&m m = get1(); //m 为int型,而get1返回的是void型,这在编译时会出问题 memcpy(m,"aaa",3); //由于上面get1中buf已被释放,所以这里会出现段错误 return 0; } 可以改成如下,用C++运行 #include <stdio.h> #include <string.h> char *get1(){ char *buf = new char[12]; strcpy(buf, "abc"); return buf; } void get2(int **p){ *p = new int[10]; } int main() { int *m = NULL; get2(&m); char *p = get1(); memcpy(m, "aaa", 3); delete[] p; delete[] m; printf("sss\n"); return 0; }
在第一个代码中,有如下问题:
1. 在 get1() 函数中,定义的 buf 是局部变量,当函数结束后,buf 的内存空间已经被释放,而返回的指针仍然指向这块已经释放的内存空间,在使用这个指针时可能会导致程序崩溃或产生未定义行为。因此,get1() 函数需要改成返回动态分配的内存空间。
2. 在 get2() 函数中,传递的参数 p 是一个指针,属于值传递。如果要修改指针的值,需要传递指向指针的指针。
3. 在 main() 函数中,m 是一个野指针,没有初始化。在 get2() 函数中,也没有对传入的指针进行初始化。应该将 m 初始化为 NULL,并且在 get2() 函数中传入指向指针的指针,对指针进行初始化。
4. 在 main() 函数中,m 是一个 int 类型的指针,而 get1() 函数返回的是 void 类型的指针,这会导致编译错误。应该将 get1() 函数改成返回 char* 类型的指针。
5. 在 main() 函数中,使用 memcpy() 函数将 "aaa" 复制到 m 所指向的内存空间中,但是在 get1() 函数中,buf 已经被释放,这样会导致段错误。应该先将 get1() 函数返回的指针保存到一个变量中,然后再进行操作。
以下是修正后的代码:
```cpp
#include <stdio.h>
#include <string.h>
char *get1() {
char *buf = new char[12];
strcpy(buf, "abc");
return buf;
}
void get2(int **p) {
*p = new int[10];
}
int main() {
int *m = NULL;
get2(&m);
char *p = get1();
memcpy(m, "aaa", 3);
delete[] p;
delete[] m;
printf("sss\n");
return 0;
}
```
相关推荐
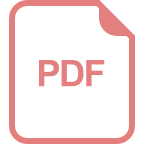
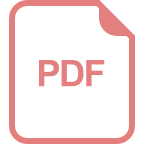
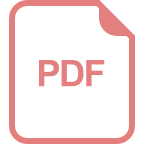













