编写一个程序,程序实现对用户指定的文本文件中的英文字符和字符串的个数进行统计的功能,并将结果根据用户选择输出至结果文件或屏幕。 构建统计类,该类实现对I/O的操纵;实现对文本文件中英文字符、字符串的统计;实现对统计结果的输出。 构建测试类,该类实现与用户的交互,向用户提示操作信息,并接收用户的操作请求。
时间: 2024-02-24 21:53:30 浏览: 23
下面是一个 Java 实现的程序,实现对用户指定的文本文件中的英文字符和字符串的个数进行统计的功能,并将结果根据用户选择输出至结果文件或屏幕。
统计类 TextStatistics:
```java
import java.io.*;
public class TextStatistics {
private String inputFileName;
private String outputFileName;
private int numChars;
private int numWords;
public TextStatistics(String inputFile, String outputFile) {
inputFileName = inputFile;
outputFileName = outputFile;
numChars = 0;
numWords = 0;
}
public void count() {
try {
BufferedReader reader = new BufferedReader(new FileReader(inputFileName));
String line;
while ((line = reader.readLine()) != null) {
numChars += line.length();
String[] words = line.split("\\W+");
numWords += words.length;
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void output(boolean toFile) {
String output = "Number of characters: " + numChars + "\n";
output += "Number of words: " + numWords + "\n";
if (toFile) {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(outputFileName));
writer.write(output);
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println(output);
}
}
}
```
测试类 TextStatisticsTest:
```java
import java.util.Scanner;
public class TextStatisticsTest {
public static void main(String[] args) {
System.out.println("Welcome to Text Statistics!");
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter the name of the input file: ");
String inputFile = scanner.nextLine();
System.out.println("Please enter the name of the output file: ");
String outputFile = scanner.nextLine();
System.out.println("Do you want to output to a file? (Y/N)");
boolean toFile = scanner.nextLine().equalsIgnoreCase("Y");
TextStatistics stats = new TextStatistics(inputFile, outputFile);
stats.count();
stats.output(toFile);
System.out.println("Text statistics finished!");
}
}
```
程序使用 BufferedReader 和 FileWriter 类实现对文本文件的读取和写入,使用 split() 方法实现对字符串的切割,同时使用正则表达式 "\\W+" 去除非字母数字字符。用户可以通过控制台输入输入文件名、输出文件名和是否输出到文件的选项,并调用 TextStatistics 类的方法进行统计和输出。
相关推荐
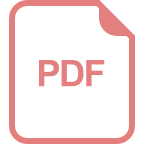
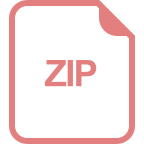
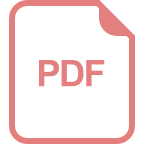
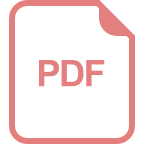
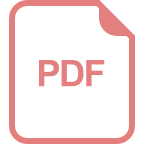
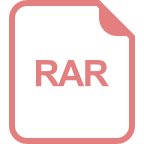
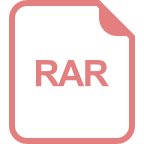
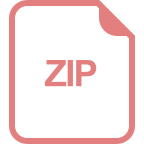
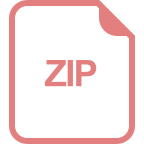
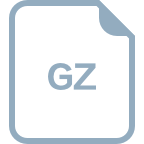
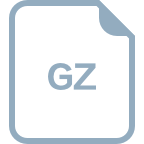
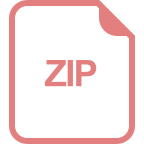
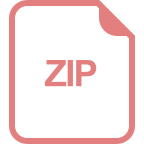