用Java swing写一个代码,做一个超市商品结算界面并可以通过坐标调整,读取文件1.txt,文件包括商品名,商品数量,商品价格,可以通过搜索商品名称,显示该商品的信息,点击添加键,则将选择的商品展示在小的文本框,包括商品名,商品数量,商品价格,其中商品数量可以进行修改,并且计算出所有商品总价,点击结算按钮后,跳转至新界面
时间: 2024-04-18 16:33:33 浏览: 139
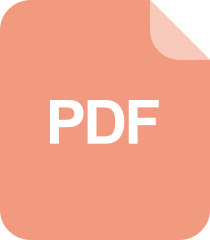
Java编写网上超市购物结算功能程序

以下是一个简单的Java Swing代码示例,实现了超市商品结算界面的功能:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class SupermarketCheckout {
private JFrame frame;
private JTextField searchField, nameField, quantityField, priceField;
private JTextArea cartArea;
private JLabel totalPriceLabel;
private JButton addButton, checkoutButton;
private double totalPrice;
public SupermarketCheckout() {
frame = new JFrame("Supermarket Checkout");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(500, 400);
frame.setLayout(new BorderLayout());
JPanel topPanel = new JPanel();
topPanel.setLayout(new FlowLayout());
JLabel searchLabel = new JLabel("Search:");
searchField = new JTextField(15);
JButton searchButton = new JButton("Search");
searchButton.addActionListener(new SearchButtonListener());
topPanel.add(searchLabel);
topPanel.add(searchField);
topPanel.add(searchButton);
JPanel centerPanel = new JPanel();
centerPanel.setLayout(new GridLayout(4, 2));
JLabel nameLabel = new JLabel("Name:");
nameField = new JTextField(15);
nameField.setEditable(false);
JLabel quantityLabel = new JLabel("Quantity:");
quantityField = new JTextField(15);
JLabel priceLabel = new JLabel("Price:");
priceField = new JTextField(15);
priceField.setEditable(false);
addButton = new JButton("Add");
addButton.setEnabled(false);
addButton.addActionListener(new AddButtonListener());
centerPanel.add(nameLabel);
centerPanel.add(nameField);
centerPanel.add(quantityLabel);
centerPanel.add(quantityField);
centerPanel.add(priceLabel);
centerPanel.add(priceField);
centerPanel.add(addButton);
JPanel bottomPanel = new JPanel();
bottomPanel.setLayout(new BorderLayout());
JLabel cartLabel = new JLabel("Cart:");
cartArea = new JTextArea(10, 30);
cartArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(cartArea);
JPanel totalPricePanel = new JPanel();
totalPricePanel.setLayout(new FlowLayout(FlowLayout.RIGHT));
JLabel totalPriceTextLabel = new JLabel("Total Price:");
totalPriceLabel = new JLabel("0.0");
totalPricePanel.add(totalPriceTextLabel);
totalPricePanel.add(totalPriceLabel);
bottomPanel.add(cartLabel, BorderLayout.NORTH);
bottomPanel.add(scrollPane, BorderLayout.CENTER);
bottomPanel.add(totalPricePanel, BorderLayout.SOUTH);
checkoutButton = new JButton("Checkout");
checkoutButton.addActionListener(new CheckoutButtonListener());
frame.add(topPanel, BorderLayout.NORTH);
frame.add(centerPanel, BorderLayout.CENTER);
frame.add(bottomPanel, BorderLayout.SOUTH);
frame.add(checkoutButton, BorderLayout.EAST);
frame.setVisible(true);
}
private void searchProduct(String name) {
try {
Scanner scanner = new Scanner(new File("1.txt"));
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] parts = line.split(",");
if (parts[0].equals(name)) {
nameField.setText(parts[0]);
quantityField.setText(parts[1]);
priceField.setText(parts[2]);
addButton.setEnabled(true);
return;
}
}
// If product not found
nameField.setText("");
quantityField.setText("");
priceField.setText("");
addButton.setEnabled(false);
JOptionPane.showMessageDialog(frame, "Product not found!");
} catch (FileNotFoundException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(frame, "File not found!");
}
}
private void addToCart() {
String name = nameField.getText();
int quantity = Integer.parseInt(quantityField.getText());
double price = Double.parseDouble(priceField.getText());
double subtotal = quantity * price;
cartArea.append(name + " x " + quantity + " - $" + subtotal + "\n");
totalPrice += subtotal;
totalPriceLabel.setText(String.valueOf(totalPrice));
}
private void checkout() {
frame.dispose();
JFrame checkoutFrame = new JFrame("Checkout");
checkoutFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
checkoutFrame.setSize(300, 200);
checkoutFrame.setLayout(new FlowLayout());
JLabel thankYouLabel = new JLabel("Thank you for shopping!");
JLabel totalLabel = new JLabel("Total Price: $" + totalPrice);
checkoutFrame.add(thankYouLabel);
checkoutFrame.add(totalLabel);
checkoutFrame.setVisible(true);
}
private class SearchButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
String productName = searchField.getText();
searchProduct(productName);
}
}
private class AddButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
addToCart();
}
}
private class CheckoutButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
checkout();
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new SupermarketCheckout();
}
});
}
}
```
请确保在运行代码之前,将名为`1.txt`的商品信息文件放置在与代码文件相同的目录下,并按照以下格式编写文件内容:
```
商品1,数量1,价格1
商品2,数量2,价格2
...
```
运行代码后,将会弹出一个窗口显示超市商品结算界面。你可以输入商品名称并点击搜索按钮来查找商品信息。找到商品后,它的名称、数量和价格将显示在相应的文本框中。你可以修改数量并点击“添加”按钮将商品添加到购物车中。购物车中的商品将显示在文本区域中,并计算出总价。当你点击“结算”按钮时,将会跳转到一个新的界面显示感谢信息和总价。
请注意,这只是一个简单的示例,可能需要根据具体需求进行进一步的修改和完善。
阅读全文
相关推荐
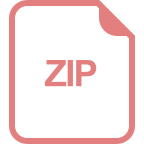
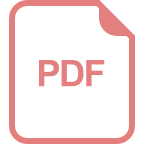
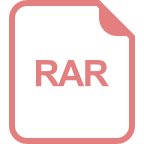
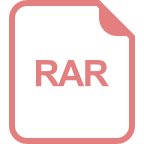
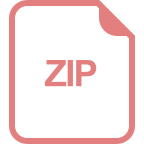
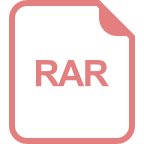
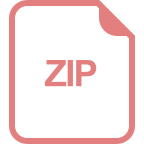
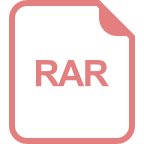
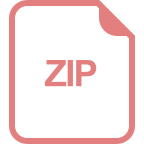
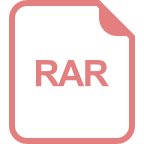
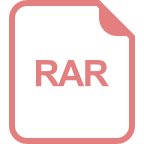
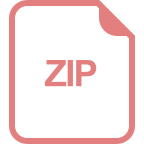
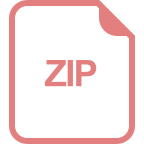
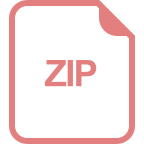
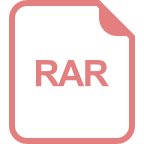
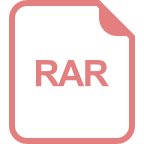
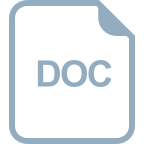