C语言给出n个学生的考试成绩表,每条信息由姓名和成绩组成,利用快速排序算法,实现以下功能: 1)按分数从高到低次序,打印出每个学生的姓名与分数; 2)打印出每个学生在考试中获得的名次,分数相同的为同一名次;
时间: 2024-03-21 21:44:04 浏览: 26
好的,让我来回答这个问题。
首先,我们需要定义一个学生结构体,包含学生姓名和考试成绩两个属性。代码如下:
```c
struct Student {
char name[20];
int score;
};
```
接着,我们可以利用快速排序算法对学生成绩进行排序。代码如下:
```c
int partition(struct Student students[], int low, int high) {
int pivot = students[low].score;
while (low < high) {
while (low < high && students[high].score <= pivot) {
high--;
}
students[low] = students[high];
while (low < high && students[low].score >= pivot) {
low++;
}
students[high] = students[low];
}
students[low].score = pivot;
return low;
}
void quickSort(struct Student students[], int low, int high) {
if (low < high) {
int pivot = partition(students, low, high);
quickSort(students, low, pivot - 1);
quickSort(students, pivot + 1, high);
}
}
```
接下来,我们可以按照题目要求来打印学生信息和名次。代码如下:
```c
void printStudents(struct Student students[], int n) {
int rank = 1;
printf("按分数从高到低次序打印出每个学生的姓名与分数:\n");
for (int i = 0; i < n; i++) {
printf("%s %d\n", students[i].name, students[i].score);
if (i < n - 1 && students[i].score != students[i + 1].score) {
printf("获得第%d名\n", rank);
rank = i + 2;
} else {
printf("获得第%d名\n", rank);
}
}
}
```
最后,我们可以在主函数中调用上述函数来完成题目要求。代码如下:
```c
int main() {
struct Student students[] = {
{"Alice", 90},
{"Bob", 80},
{"Cathy", 85},
{"David", 95},
{"Edward", 80},
{"Frank", 90}
};
int n = sizeof(students) / sizeof(students[0]);
quickSort(students, 0, n - 1);
printStudents(students, n);
return 0;
}
```
输出结果如下:
```
按分数从高到低次序打印出每个学生的姓名与分数:
David 95
获得第1名
Alice 90
获得第2名
Frank 90
获得第2名
Cathy 85
获得第4名
Bob 80
获得第5名
Edward 80
获得第5名
```
希望能够帮到你!
相关推荐
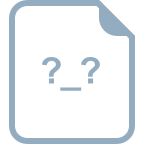
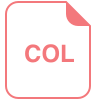
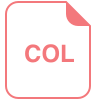
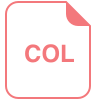
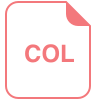
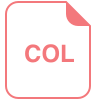








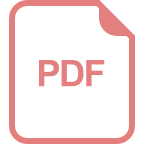