生成使用mean shift算法进行图像聚类分割的matlab代码
时间: 2023-06-15 16:07:30 浏览: 275
以下是使用mean shift算法进行图像聚类分割的matlab代码:
```matlab
clc;clear;close all;
% 读取图像
I = imread('test.jpg');
figure,imshow(I);
% 将图像转化为二维矩阵
[m,n,k] = size(I);
X = double(reshape(I,m*n,k));
% 计算带宽
bandwidth = 30;
% 设置停止条件
tol = 1e-3;
% 计算mean shift向量
ms = ms_func(X,bandwidth,tol);
% 计算聚类结果
[clustCent,data2cluster,cluster2dataCell] = MeanShiftCluster(X',bandwidth,ms);
numClust = length(cluster2dataCell);
% 显示聚类结果
figure,hold on
colors = 'rgbk';
for i = 1:numClust
index = cluster2dataCell{i};
scatter3(X(index,1),X(index,2),X(index,3),35,colors(mod(i,4)+1));
end
hold off
```
其中,ms_func函数如下所示:
```matlab
function ms = ms_func(X,bandwidth,tol)
% 初始化mean shift向量
ms = zeros(size(X));
% 开始迭代
for i = 1:size(X,1)
x = X(i,:)';
prev_x = x;
diff = inf;
iter = 0;
while norm(diff) > tol
% 计算当前点的mean shift向量
[idx, dist] = rangesearch(X,x',bandwidth);
idx = idx{1};
weight = exp(-(dist{1}/bandwidth).^2);
ms_x = sum(bsxfun(@times,X(idx,:),weight),1)/sum(weight);
% 计算与上一个点的差异
diff = ms_x' - x;
x = ms_x';
% 更新迭代次数
iter = iter + 1;
if iter > 100
break;
end
end
% 保存mean shift向量
ms(i,:) = x;
end
end
```
MeanShiftCluster函数如下所示:
```matlab
function [clustCent,data2cluster,cluster2dataCell] = MeanShiftCluster(dataPts,bandwidth,ms)
% 获取数据点数量
numPoints = size(dataPts,2);
% 初始化标记矩阵
beenVisitedFlag = zeros(1,numPoints,'uint8');
clusterIndex = 1;
numClusters = 0;
clusterVotes = zeros(1,numPoints,'uint16');
% 开始聚类
while (sum(beenVisitedFlag) < numPoints)
% 找到未被访问的点
index = find(beenVisitedFlag == 0,1);
thisClusterVotes = zeros(1,numPoints,'uint16');
% 找到该点的簇
while true
% 计算当前点与所有点的距离
dists = sqrt(sum(bsxfun(@minus,ms(index,:),ms).^2,2));
% 找到与当前点距离小于等于带宽的点
inCluster = find(dists <= bandwidth);
% 统计该簇的投票数
thisClusterVotes(inCluster) = thisClusterVotes(inCluster) + 1;
% 更新已访问标记
beenVisitedFlag(inCluster) = 1;
% 如果该簇中的点数量大于等于3,则认为该簇合法
if length(inCluster) >= 3
break;
end
% 如果该簇中的点数量小于3,则找到最近的点,继续迭代
idx = find(beenVisitedFlag == 0);
dists = dists(idx);
[minDist,loc] = min(dists);
index = idx(loc);
end
% 更新聚类中心
clustCent(clusterIndex,:) = mean(ms(inCluster,:),1);
% 记录该簇的投票数
clusterVotes = clusterVotes + thisClusterVotes;
% 将该簇的所有点加入簇集合中
data2cluster(inCluster) = clusterIndex;
% 更新簇集合
cluster2dataCell{clusterIndex} = inCluster;
numClusters = numClusters + 1;
clusterIndex = clusterIndex + 1;
end
end
```
运行代码后,将生成聚类后的图像。
阅读全文
相关推荐
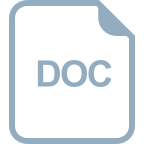
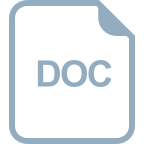
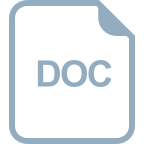
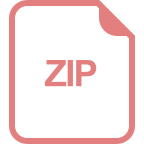

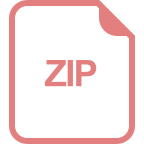
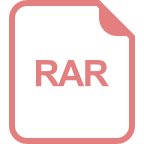
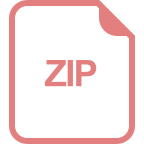
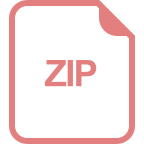
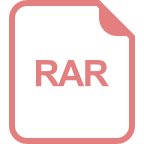
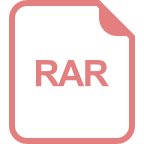
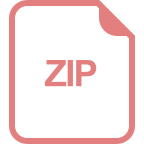
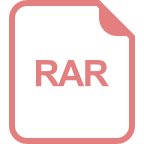





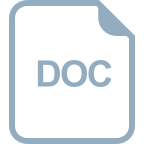