myeclipse中JDBC连接mysql和简单的增删改查
时间: 2023-09-18 12:09:15 浏览: 81
在 MyEclipse 中进行 JDBC 连接 MySQL 可以分为以下几个步骤:
1. 导入 MySQL 驱动包
将 MySQL 的 JDBC 驱动包(mysql-connector-java.jar)复制到 MyEclipse 项目的 lib 目录下,然后右键单击该驱动包,选择 Build Path -> Add to Build Path 将其添加到项目的构建路径中。
2. 编写 JDBC 连接代码
在 Java 代码中编写 JDBC 连接代码,示例代码如下:
```java
import java.sql.*;
public class JdbcTest {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 1. 加载数据库驱动
Class.forName("com.mysql.jdbc.Driver");
// 2. 获取数据库连接
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 3. 创建 Statement 对象
stmt = conn.createStatement();
// 4. 执行 SQL 查询
String sql = "select * from user";
rs = stmt.executeQuery(sql);
// 5. 处理查询结果
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
System.out.println("id=" + id + ", name=" + name);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 6. 关闭资源
try { rs.close(); } catch (Exception e) {}
try { stmt.close(); } catch (Exception e) {}
try { conn.close(); } catch (Exception e) {}
}
}
}
```
3. 运行程序
在 MyEclipse 中运行该程序,如果一切正常,就会输出查询结果。
至于简单的增删改查操作,可以使用 PreparedStatement 对象来执行 SQL 语句。示例代码如下:
```java
import java.sql.*;
public class JdbcTest {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
// 1. 加载数据库驱动
Class.forName("com.mysql.jdbc.Driver");
// 2. 获取数据库连接
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 3. 执行插入操作
String sql = "insert into user (name, age) values (?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "张三");
pstmt.setInt(2, 18);
pstmt.executeUpdate();
// 4. 执行更新操作
sql = "update user set age = ? where name = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, 20);
pstmt.setString(2, "张三");
pstmt.executeUpdate();
// 5. 执行删除操作
sql = "delete from user where name = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "张三");
pstmt.executeUpdate();
// 6. 执行查询操作
sql = "select * from user";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("id=" + id + ", name=" + name + ", age=" + age);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 7. 关闭资源
try { rs.close(); } catch (Exception e) {}
try { pstmt.close(); } catch (Exception e) {}
try { conn.close(); } catch (Exception e) {}
}
}
}
```
以上代码实现了插入一条记录、更新一条记录、删除一条记录和查询所有记录的操作。
相关推荐
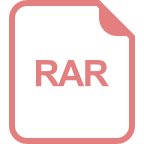














