python写数据库查询页面
时间: 2023-08-10 08:33:21 浏览: 158
要使用Python编写数据库查询页面,需要先安装数据库驱动程序和Web框架。下面以MySQL数据库和Flask Web框架为例,简单介绍实现步骤。
1. 安装MySQL驱动程序和Flask框架
在Python环境下使用pip命令安装MySQL驱动程序和Flask框架:
```
pip install mysql-connector-python flask
```
2. 创建Flask应用
在项目目录下创建一个名为app.py的Python文件,输入以下代码:
```python
from flask import Flask, render_template, request
import mysql.connector
app = Flask(__name__)
# 数据库连接配置
config = {
'user': 'root',
'password': '123456',
'host': 'localhost',
'database': 'test'
}
# 查询页面路由
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
keyword = request.form['keyword']
conn = mysql.connector.connect(**config)
cursor = conn.cursor()
sql = "SELECT * FROM users WHERE name LIKE '%{}%'".format(keyword)
cursor.execute(sql)
results = cursor.fetchall()
cursor.close()
conn.close()
return render_template('index.html', results=results)
else:
return render_template('index.html')
if __name__ == '__main__':
app.run()
```
以上代码定义了一个名为app的Flask应用实例,创建了一个查询页面的路由函数index(),该函数处理查询请求并返回查询结果。
3. 创建HTML模板
在项目目录下创建一个名为templates的文件夹,在该文件夹下创建一个名为index.html的HTML模板文件,输入以下代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>查询页面</title>
</head>
<body>
<form action="/" method="post">
<label for="keyword">关键字:</label>
<input type="text" id="keyword" name="keyword">
<input type="submit" value="查询">
</form>
<table border="1">
<tr>
<th>姓名</th>
<th>性别</th>
<th>年龄</th>
</tr>
{% for result in results %}
<tr>
<td>{{ result[1] }}</td>
<td>{{ result[2] }}</td>
<td>{{ result[3] }}</td>
</tr>
{% endfor %}
</table>
</body>
</html>
```
以上代码定义了一个查询表单和一个用于显示查询结果的表格,使用了Flask提供的模板引擎Jinja2。
4. 运行Flask应用
在命令行中输入以下命令,启动Flask应用:
```
python app.py
```
在浏览器中访问http://localhost:5000,即可看到查询页面。输入关键字并点击查询按钮,即可显示查询结果。
以上就是使用Python编写数据库查询页面的基本步骤。
阅读全文
相关推荐
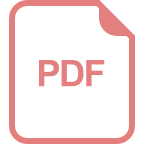
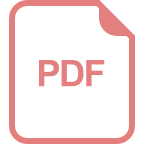
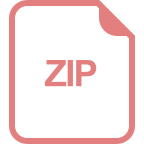
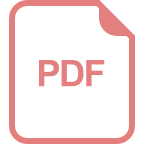
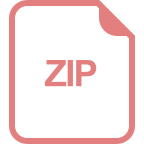
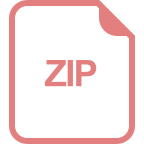
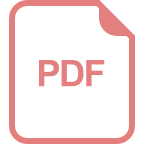
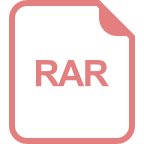
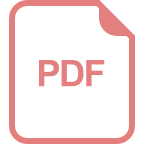
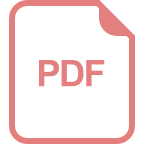
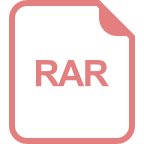
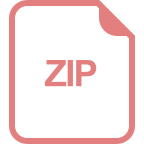
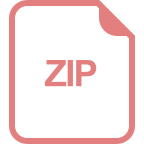
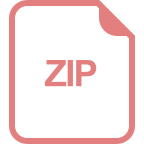
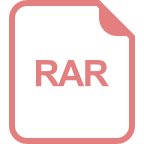
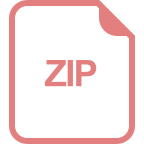