用c语言写一个环形链表
时间: 2023-03-16 08:43:48 浏览: 158
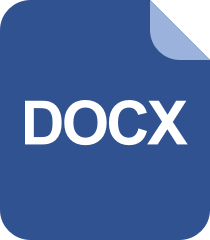
C语言实现链表

我可以为您提供一个简单的示例:#include <stdio.h>
#include <stdlib.h> // 环形链表节点
struct Node
{
int data;
struct Node *next;
}; // 在头结点之后插入新节点
void insert(struct Node **head_ref, int data)
{
// 分配内存
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
struct Node *last = *head_ref;
// 把新节点的数据和指针赋值
new_node->data = data;
new_node->next = NULL;
// 如果头结点是空的,把头结点设为新节点
if (*head_ref == NULL)
{
*head_ref = new_node;
return;
}
// 否则,找到最后一个节点
while (last->next != *head_ref)
last = last->next;
// 把新节点添加到最后一个节点之后
last->next = new_node;
// 把最后一个节点指向头结点
new_node->next = *head_ref;
} // 打印环形链表
void printList(struct Node *head)
{
struct Node *temp = head;
if (head != NULL)
{
do
{
printf("%d ", temp->data);
temp = temp->next;
}
while (temp != head);
}
} // 主函数
int main()
{
// 创建一个环形链表
struct Node *head = NULL;
// 插入4个节点
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
insert(&head, 4);
// 打印环形链表
printList(head);
return 0;
}
阅读全文
相关推荐
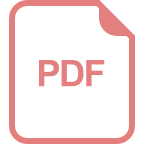
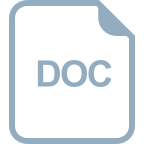









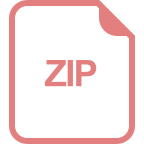




