kotlin编写一个包含String和Int参数的工具类
时间: 2024-05-14 16:16:48 浏览: 21
好的,关于你提出的问题,我可以给出以下 Kotlin 代码示例,实现一个包含 String 和 Int 参数的工具类:
```
class MyUtils {
companion object {
fun combineStringAndInt(s: String, i: Int): String {
return "$s : $i" // 将字符串和整数参数拼接并返回
}
}
}
```
在以上代码中,我们定义了一个包含 `combineStringAndInt` 静态方法的 Kotlin 工具类,该方法接收一个 String 和一个 Int 类型的参数,并将这两个参数拼接为一个新的字符串,返回给调用者。
需要注意的是,我们将 `combineStringAndInt` 方法定义在 `companion object`(伴随对象)中,这样可以不需要实例化对象,直接通过类名调用该方法。
希望以上示例代码对您有帮助。
相关问题
MD5加密工具类kotlin
MD5加密工具类可以在Kotlin中使用以下代码实现:
fun encode(text: String): String {
try {
// 获取MD5加密对象
val instance: MessageDigest = MessageDigest.getInstance("MD5")
// 对字符串加密,返回字节数组
val digest: ByteArray = instance.digest(text.toByteArray())
var sb: StringBuffer = StringBuffer()
for (b in digest) {
// 获取低八位有效值
var i: Int = b.toInt() and 0xff
// 将整数转化为16进制
var hexString = Integer.toHexString(i)
if (hexString.length < 2) {
// 如果是一位的话,补0
hexString = "0$hexString"
}
sb.append(hexString)
}
return sb.toString()
} catch (e: NoSuchAlgorithmException) {
e.printStackTrace()
}
return ""
}
这个方法可以直接定义为顶级函数,在需要的地方直接使用,例如:
val encryptedText = encode(password)<span class="em">1</span><span class="em">2</span><span class="em">3</span>
写kotlin代码 功能为 加载数据为空时的占位图支持点击刷新的工具类
好的,我会尽力来回答你的问题。以下是一个样例 Kotlin 工具类,用于在数据加载为空时显示占位图,并支持点击刷新:
```kotlin
import android.content.Context
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.ImageView
import android.widget.TextView
class EmptyStateUtils(private val context: Context) {
fun showEmptyState(
parent: ViewGroup,
message: String,
imageRes: Int,
clickListener: (() -> Unit)? = null
) {
val emptyStateView = LayoutInflater.from(context).inflate(R.layout.layout_empty_state, parent, false)
parent.addView(emptyStateView)
val messageTextView = emptyStateView.findViewById<TextView>(R.id.empty_state_message)
val imageView = emptyStateView.findViewById<ImageView>(R.id.empty_state_image)
messageTextView.text = message
imageView.setImageResource(imageRes)
if (clickListener != null) {
emptyStateView.setOnClickListener { clickListener.invoke() }
}
}
fun hideEmptyState(parent: ViewGroup) {
parent.removeAllViews()
}
}
```
此工具类使用 LayoutInflater 从一个指定的 layout 文件中创建了一个占位图视图。当需要显示占位图时,我们可以调用 showEmptyState() 函数,该函数将占位图添加到指定的 ViewGroup 中,同时设置了占位图的文本和图像资源。如果需要支持点击刷新,则可以传入一个回调函数。
当不再需要占位图时,我们可以调用 hideEmptyState() 函数,该函数将从指定的 ViewGroup 中移除占位图视图。
此工具类的占位图布局可以通过 layout_empty_state.xml 文件来定义:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/empty_state_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/empty_state_image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_empty_state" />
<TextView
android:id="@+id/empty_state_message"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="No data available." />
</LinearLayout>
```
当然,你可以根据自己的需求来修改布局文件。希望这个样例代码能对你有所帮助!
相关推荐
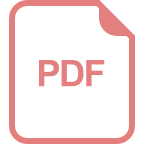
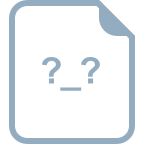
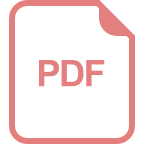
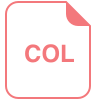
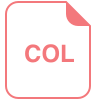
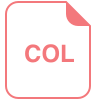
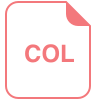
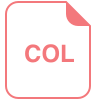







