java连接数据库做简单图书管理系统
时间: 2023-07-20 14:28:48 浏览: 60
好的,下面是一个简单的Java连接数据库并实现图书管理系统的示例代码:
1. 首先需要在MySQL数据库中创建一个名为"library"的数据库,并在其中创建一个名为"books"的表,包括以下字段:id、title、author、publisher、price。
2. 在Java中引入MySQL的JDBC驱动包,以便连接数据库。可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.19</version>
</dependency>
```
3. 在Java代码中连接数据库,可以使用以下代码:
```java
import java.sql.*;
public class DatabaseConnection {
private Connection conn;
public DatabaseConnection() {
String driver = "com.mysql.cj.jdbc.Driver";
String url = "jdbc:mysql://localhost:3306/library?useSSL=false&serverTimezone=UTC";
String username = "root";
String password = "password";
try {
Class.forName(driver);
conn = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
public Connection getConnection() {
return conn;
}
public void closeConnection() {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
4. 在Java中实现图书管理系统的功能,可以使用以下代码:
```java
import java.sql.*;
public class LibraryManagement {
private DatabaseConnection dbConn;
public LibraryManagement() {
dbConn = new DatabaseConnection();
}
public void addBook(Book book) {
Connection conn = dbConn.getConnection();
String sql = "INSERT INTO books(title, author, publisher, price) VALUES (?, ?, ?, ?)";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getPublisher());
pstmt.setDouble(4, book.getPrice());
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void updateBook(Book book) {
Connection conn = dbConn.getConnection();
String sql = "UPDATE books SET title=?, author=?, publisher=?, price=? WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getPublisher());
pstmt.setDouble(4, book.getPrice());
pstmt.setInt(5, book.getId());
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void deleteBook(int id) {
Connection conn = dbConn.getConnection();
String sql = "DELETE FROM books WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
pstmt.executeUpdate();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public Book findBookById(int id) {
Connection conn = dbConn.getConnection();
String sql = "SELECT * FROM books WHERE id=?";
try {
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
Book book = new Book();
book.setId(rs.getInt("id"));
book.setTitle(rs.getString("title"));
book.setAuthor(rs.getString("author"));
book.setPublisher(rs.getString("publisher"));
book.setPrice(rs.getDouble("price"));
rs.close();
pstmt.close();
return book;
}
rs.close();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
public List<Book> findAllBooks() {
Connection conn = dbConn.getConnection();
String sql = "SELECT * FROM books";
List<Book> books = new ArrayList<>();
try {
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
Book book = new Book();
book.setId(rs.getInt("id"));
book.setTitle(rs.getString("title"));
book.setAuthor(rs.getString("author"));
book.setPublisher(rs.getString("publisher"));
book.setPrice(rs.getDouble("price"));
books.add(book);
}
rs.close();
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
return books;
}
public void close() {
dbConn.closeConnection();
}
}
```
其中Book类的代码如下:
```java
public class Book {
private int id;
private String title;
private String author;
private String publisher;
private double price;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
使用示例:
```java
LibraryManagement lm = new LibraryManagement();
lm.addBook(new Book("Java编程思想", "Bruce Eckel", "机械工业出版社", 89.00));
lm.addBook(new Book("Java核心技术", "Cay S. Horstmann", "机械工业出版社", 99.00));
lm.updateBook(new Book(1, "Java编程思想(第4版)", "Bruce Eckel", "机械工业出版社", 99.00));
lm.deleteBook(2);
Book book = lm.findBookById(1);
System.out.println(book.getTitle());
List<Book> books = lm.findAllBooks();
for (Book b : books) {
System.out.println(b.getTitle());
}
lm.close();
```
相关推荐
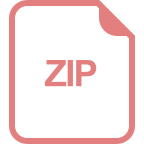














