使用OpenGL画出一个带有光照的茶壶,并且能够用键盘进行平移缩放和旋转
时间: 2024-05-13 22:16:11 浏览: 25
下面是一个使用OpenGL画出带有光照的茶壶的示例代码。该程序能够响应键盘事件进行平移缩放和旋转。
```cpp
#include <GL/glut.h>
GLfloat light_position[] = { 1.0, 1.0, 1.0, 0.0 };
GLfloat light_ambient[] = { 0.0, 0.0, 0.0, 1.0 };
GLfloat light_diffuse[] = { 1.0, 1.0, 1.0, 1.0 };
GLfloat light_specular[] = { 1.0, 1.0, 1.0, 1.0 };
GLfloat mat_ambient[] = { 0.7, 0.7, 0.7, 1.0 };
GLfloat mat_diffuse[] = { 0.8, 0.8, 0.8, 1.0 };
GLfloat mat_specular[] = { 1.0, 1.0, 1.0, 1.0 };
GLfloat mat_shininess[] = { 100.0 };
GLfloat xrot = 0.0, yrot = 0.0, zrot = 0.0;
GLfloat xscale = 1.0, yscale = 1.0, zscale = 1.0;
GLfloat xtrans = 0.0, ytrans = 0.0, ztrans = 0.0;
void init(void)
{
glEnable(GL_DEPTH_TEST);
glLightfv(GL_LIGHT0, GL_POSITION, light_position);
glLightfv(GL_LIGHT0, GL_AMBIENT, light_ambient);
glLightfv(GL_LIGHT0, GL_DIFFUSE, light_diffuse);
glLightfv(GL_LIGHT0, GL_SPECULAR, light_specular);
glEnable(GL_LIGHTING);
glEnable(GL_LIGHT0);
glMaterialfv(GL_FRONT, GL_AMBIENT, mat_ambient);
glMaterialfv(GL_FRONT, GL_DIFFUSE, mat_diffuse);
glMaterialfv(GL_FRONT, GL_SPECULAR, mat_specular);
glMaterialfv(GL_FRONT, GL_SHININESS, mat_shininess);
}
void display(void)
{
glClearColor(0.0, 0.0, 0.0, 0.0);
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glTranslatef(xtrans, ytrans, ztrans);
glScalef(xscale, yscale, zscale);
glRotatef(xrot, 1.0, 0.0, 0.0);
glRotatef(yrot, 0.0, 1.0, 0.0);
glRotatef(zrot, 0.0, 0.0, 1.0);
glutSolidTeapot(1.0);
glPopMatrix();
glutSwapBuffers();
}
void reshape(int w, int h)
{
glViewport(0, 0, (GLsizei)w, (GLsizei)h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(60.0, (GLfloat)w / (GLfloat)h, 1.0, 20.0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glTranslatef(0.0, 0.0, -5.0);
}
void keyboard(unsigned char key, int x, int y)
{
switch (key)
{
case 'x':
xrot += 5.0;
break;
case 'X':
xrot -= 5.0;
break;
case 'y':
yrot += 5.0;
break;
case 'Y':
yrot -= 5.0;
break;
case 'z':
zrot += 5.0;
break;
case 'Z':
zrot -= 5.0;
break;
case 's':
xscale *= 0.9;
yscale *= 0.9;
zscale *= 0.9;
break;
case 'S':
xscale /= 0.9;
yscale /= 0.9;
zscale /= 0.9;
break;
case 't':
xtrans += 0.1;
break;
case 'T':
xtrans -= 0.1;
break;
case 'u':
ytrans += 0.1;
break;
case 'U':
ytrans -= 0.1;
break;
case 'v':
ztrans += 0.1;
break;
case 'V':
ztrans -= 0.1;
break;
case 27:
exit(0);
break;
default:
break;
}
glutPostRedisplay();
}
int main(int argc, char **argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(500, 500);
glutInitWindowPosition(100, 100);
glutCreateWindow("Teapot with Light");
init();
glutDisplayFunc(display);
glutReshapeFunc(reshape);
glutKeyboardFunc(keyboard);
glutMainLoop();
return 0;
}
```
在这个程序中,我们使用 `glutSolidTeapot()` 函数画出了一个茶壶。我们使用 `glTranslatef()`、`glRotatef()` 和 `glScalef()` 函数实现平移、旋转和缩放操作。我们使用 `gluPerspective()` 函数设置透视投影。我们使用 `glutKeyboardFunc()` 函数响应键盘事件。
相关推荐
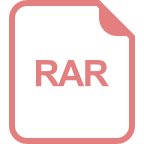
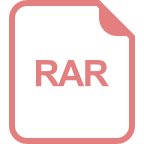
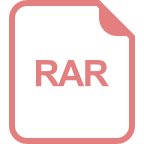
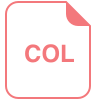
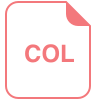












