应用jquery,servlet,ajax 实现无刷新的,每次从数据库中获取一次最新公告,并滚动显示。前端和后端代码
时间: 2024-03-09 16:45:34 浏览: 23
前端代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>公告滚动显示</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<style type="text/css">
#notice {
width: 300px;
height: 50px;
overflow: hidden;
font-size: 18px;
line-height: 50px;
text-align: center;
border: 1px solid #ccc;
}
</style>
</head>
<body>
<div id="notice"></div>
<script>
$(function() {
setInterval(getNotice, 5000); // 5秒钟获取一次公告
});
function getNotice() {
$.ajax({
type: "POST",
url: "GetNoticeServlet",
dataType: "json",
success: function(result) {
if (result.success) {
var notice = result.data;
$('#notice').text(notice);
$('#notice').slideDown();
setTimeout(function() {
$('#notice').slideUp();
}, 3000);
}
}
});
}
</script>
</body>
</html>
```
后端代码(Java Servlet):
```java
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.json.JSONObject;
@WebServlet("/GetNoticeServlet")
public class GetNoticeServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
private static final String DB_URL = "jdbc:mysql://localhost:3306/test";
private static final String USER = "root";
private static final String PASS = "root";
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("application/json;charset=UTF-8");
PrintWriter out = response.getWriter();
JSONObject json = new JSONObject();
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
String sql = "SELECT notice FROM notice ORDER BY id DESC LIMIT 1";
stmt = conn.prepareStatement(sql);
rs = stmt.executeQuery();
if (rs.next()) {
String notice = rs.getString("notice");
json.put("success", true);
json.put("data", notice);
} else {
json.put("success", false);
json.put("msg", "暂无公告!");
}
} catch (Exception e) {
e.printStackTrace();
json.put("success", false);
json.put("msg", "获取公告失败!");
} finally {
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
out.println(json);
out.flush();
out.close();
}
}
```
该代码实现了每隔5秒从数据库中获取最新的公告并滚动显示。前端使用了jQuery库和Ajax技术,后端使用了Java Servlet来处理请求和响应。需要注意的是,数据库连接信息需要根据实际情况修改。
相关推荐
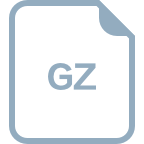














