java生成的xml里面多出来的nul如何去除
时间: 2024-09-23 22:12:37 浏览: 16
在Java中,当将数据转换为XML字符串时,有时可能会遇到额外的`null`字符,这通常是因为某些值为`null`的对象在序列化过程中被编码了。要移除XML文件中的`<null>`标签,你可以采用以下几种方法:
1. **使用DOM解析器**:
使用`javax.xml.parsers.DocumentBuilderFactory`创建一个解析器,然后选择元素并检查它们是否为`null`,如果不是再添加到XML文档中。
```java
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.newDocument();
// 假设你有一个NodeList nodes,遍历并处理每个节点
for (int i = 0; i < nodes.getLength(); i++) {
Node node = nodes.item(i);
if (node != null) {
doc.appendChild(node);
}
}
```
2. **使用JAXB (Java Architecture for XML Binding)**:
如果你正在使用JAXB,可以配置它来忽略空值。例如,在`pom.xml`中加入以下依赖,并设置属性:
```xml
<dependency>
<groupId>org.jvnet.staxex</groupId>
<artifactId>stax-ex</artifactId>
<version>1.8.1</version>
</dependency>
<build>
<plugins>
<plugin>
<groupId>org.jvnet.jaxb2.maven2</groupId>
<artifactId>maven-jaxb2-plugin</artifactId>
<configuration>
<schemaDirectory>src/main/resources/xsd/</schemaDirectory>
<generateXmlSchema>true</generateXmlSchema>
<bindingDirectory>src/main/resources/jaxb/bindings/</bindingDirectory>
<suppressMissingPropertyProblem>true</suppressMissingPropertyProblem>
<!-- ... -->
</configuration>
</plugin>
</plugins>
</build>
```
3. **自定义转换器**:
创建一个定制的`ContentHandler`或`Transformer`,在生成XML时直接跳过`null`值。
无论哪种方法,记得检查每个要序列化的值,如果为`null`,则可以选择不添加到最终的XML结构中,或者替换为默认值、空字符串或其他适当的占位符。
相关推荐
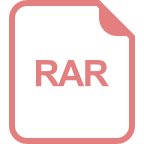
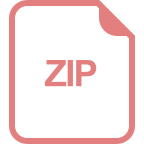
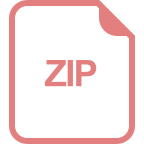














