stm32hal库学习实例:A、B、C三个终端,实现三个终端之间任意通信。要求(消息队列+父子进程) 条件:第一个:通信内容可以编辑 第二个:发送内容前几个字必须为toB,目的该消息有哪个消息接收。 第三个:当任一个进程接收到消息,必须立即显示出来消息的内容---解释:进程A输入完toC:helloipc 后,进程C在终端上显示内容:formA:helloipc
时间: 2024-04-16 19:27:47 浏览: 123
你可以使用STM32HAL库结合消息队列和父子进程来实现三个终端之间的任意通信。下面是一个示例代码,可以满足你的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_SIZE 100
// 定义消息队列的结构体
struct message {
long int msg_type;
char msg_text[MAX_SIZE];
};
int main() {
pid_t pid;
int msgid;
struct message msg;
// 创建消息队列
msgid = msgget((key_t)1234, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
// 创建子进程
pid = fork();
if (pid == -1) {
perror("fork failed");
exit(EXIT_FAILURE);
}
if (pid == 0) {
// 子进程负责接收消息并显示
while (1) {
if (msgrcv(msgid, (void *)&msg, MAX_SIZE, getpid(), 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
printf("From parent: %s\n", msg.msg_text);
}
} else {
// 父进程负责发送消息
while (1) {
char input[MAX_SIZE];
printf("Enter message to send: ");
fgets(input, MAX_SIZE, stdin);
// 将输入的消息发送给目标终端
strcpy(msg.msg_text, input);
msg.msg_type = atoi(&input[3]); // 解析目标终端号
if (msgsnd(msgid, (void *)&msg, MAX_SIZE, 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
}
}
// 删除消息队列
if (msgctl(msgid, IPC_RMID, 0) == -1) {
perror("msgctl failed");
exit(EXIT_FAILURE);
}
return 0;
}
```
以上代码创建了一个消息队列,父进程负责接收用户输入并发送消息,子进程负责接收消息并显示。父进程发送的消息格式为"toX:message"(其中X为目标终端号),子进程接收到消息后会显示"From parent:message"。
请注意,由于你提到使用了STM32HAL库,以上示例代码是在Linux环境下使用C语言编写的,可以在Linux上进行编译和运行。如果你要在STM32上实现类似功能,你需要根据STM32的开发环境和库进行相应的修改和适配。
阅读全文
相关推荐
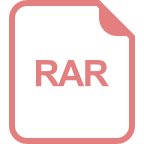
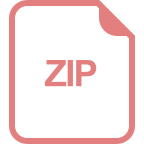
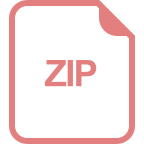
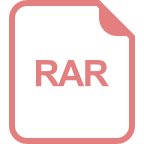
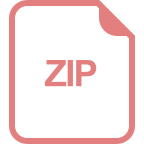
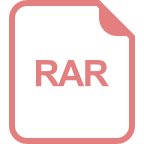
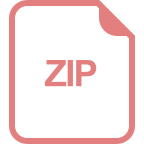
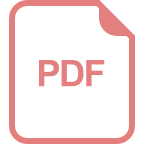
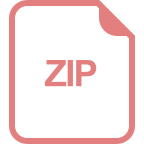
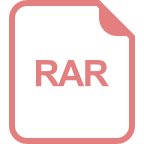
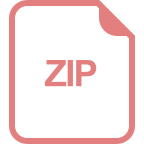
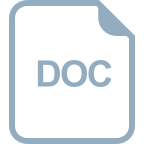
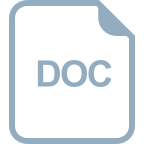