用php写出咖啡机租赁微信小程序
时间: 2024-09-22 12:02:20 浏览: 43
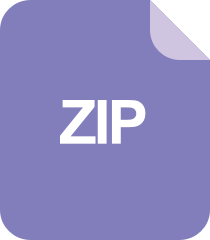
微信小程序期末大作业-手机商城微信小程序

创建一个PHP编写的咖啡机租赁微信小程序需要结合PHP后端和微信小程序的前端技术。以下是简化的步骤和部分示例代码:
**1. 创建后端框架(例如Laravel、Yii等):**
首先安装并设置一个PHP框架,如Laravel,用于处理业务逻辑和数据交互。
```php
// 使用Composer安装Laravel
composer create-project laravel/laravel coffee-machine-rental
// 进入项目目录
cd coffee-machine-rental
```
**2. 设计数据库模型:**
创建一个CoffeeMachine模型,用于存储咖啡机的信息。
```php
// CoffeeMachine.php (app/Models/CoffeeMachine.php)
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class CoffeeMachine extends Model
{
// 定义字段和关联关系
}
```
**3. 创建API接口:**
为微信小程序提供租赁、查询库存等接口。
```php
// CoffeeMachinesController.php (app/Http/Controllers/)
public function rent(CoffeeMachine $coffeeMachine) {
// 检查库存并处理租赁逻辑
}
// index action for listing machines
public function index() {
return response()->json(CoffeeMachine::all());
}
```
**4. 微信小程序前端开发:**
使用WXML、WXSS和JavaScript编写页面结构和交互逻辑。
```html
<!-- app.wxss -->
.page {
display: flex;
flex-direction: column;
}
.rent-coffee-machine {
/* ...样式... */
}
<!-- app.wxml -->
<view class="page">
<view class="rent-coffee-machine" bindtap="rentMachine">租借咖啡机</view>
<!-- 显示咖啡机列表 -->
</view>
<!-- app.js -->
Page({
rentMachine: function(e) {
wx.request({
url: 'https://your-api-url/rent', // 替换为实际API地址
data: {},
method: 'POST',
success: function(res) {
console.log(res.data);
}
});
},
})
```
**5. 配置微信小程序:**
在微信公众平台上注册并配置服务器域名,将后端API添加到合法域名白名单。
**6. 封装和部署:**
打包前端代码,并将后端部署到服务器。确保服务器支持HTTPS访问。
阅读全文
相关推荐
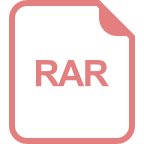
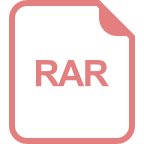
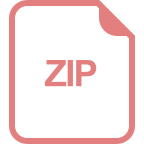
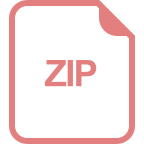
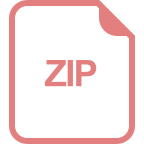
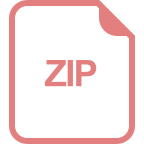
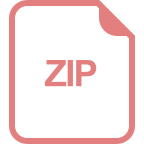
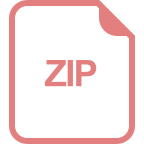
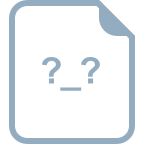
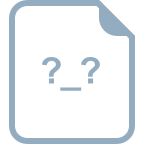
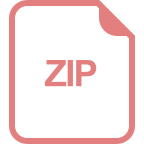
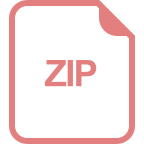
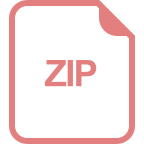