如何用C++实现一个学生成绩管理系统?
时间: 2024-11-13 20:25:36 浏览: 30
在C++中创建一个简单的学生成绩管理系统,通常会涉及到基本的数据结构如数组或向量用于存储学生信息,以及类来表示学生、课程和成绩等概念。以下是一个简化的示例:
首先,定义一个基础的学生类(Student):
```cpp
class Student {
private:
std::string name;
int id;
public:
// 构造函数
Student(const std::string& studentName, int studentId) : name(studentName), id(studentId) {}
// 获取和设置姓名、ID的方法
std::string getName() const { return name; }
void setName(const std::string& name) { this->name = name; }
int getId() const { return id; }
};
```
然后,为了管理课程和成绩,可以创建一个课程类(Course):
```cpp
class Course {
private:
std::string courseName;
std::vector<int> scores; // 使用向量存储每个学生的分数
public:
// 类的构造函数等...
void addScore(Student* student, int score) {
scores.push_back(score);
}
// 可能还需要其他方法来查询平均分等...
};
```
最后,一个主函数用于操作这个系统:
```cpp
int main() {
Course math("Math");
Course english("English");
Student student1("张三", 1001);
Student student2("李四", 1002);
math.addScore(&student1, 85);
math.addScore(&student2, 90);
english.addScore(&student1, 92);
english.addScore(&student2, 87);
// 打印学生成绩
std::cout << "学生1的数学成绩: " << math.getScores()[0] << std::endl;
std::cout << "学生1的英语成绩: " << english.getScores()[0] << std::endl;
return 0;
}
```
阅读全文
相关推荐




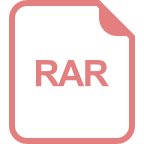










