图片数据增强python代码
时间: 2023-12-20 19:30:37 浏览: 40
以下是图片数据增强的Python代码,包括裁剪、平移、改变亮度、加噪声、旋转、镜像和cutout等方法:
```python
import cv2
import numpy as np
import random
# 裁剪
def crop(image, bbox):
x1, y1, x2, y2 = bbox
return image[y1:y2, x1:x2]
# 平移
def translate(image, bbox, x_offset, y_offset):
x1, y1, x2, y2 = bbox
M = np.float32([[1, 0, x_offset], [0, 1, y_offset]])
image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
bbox = [x1 + x_offset, y1 + y_offset, x2 + x_offset, y2 + y_offset]
return image, bbox
# 改变亮度
def brightness(image, brightness_factor):
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
hsv[:, :, 2] = hsv[:, :, 2] * brightness_factor
return cv2.cvtColor(hsv, cv2.COLOR_HSV2BGR)
# 加噪声
def noise(image):
row, col, ch = image.shape
mean = 0
var = 0.1
sigma = var ** 0.5
gauss = np.random.normal(mean, sigma, (row, col, ch))
gauss = gauss.reshape(row, col, ch)
noisy = image + gauss
return noisy
# 旋转
def rotate(image, bbox, angle):
x1, y1, x2, y2 = bbox
center = ((x1 + x2) / 2, (y1 + y2) / 2)
M = cv2.getRotationMatrix2D(center, angle, 1)
image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
x1_new, y1_new = np.dot(M, np.array([x1, y1, 1]))
x2_new, y2_new = np.dot(M, np.array([x2, y2, 1]))
bbox = [int(x1_new), int(y1_new), int(x2_new), int(y2_new)]
return image, bbox
# 镜像
def flip(image, bbox):
x1, y1, x2, y2 = bbox
image = cv2.flip(image, 1)
bbox = [image.shape[1] - x2, y1, image.shape[1] - x1, y2]
return image, bbox
# cutout
def cutout(image, bbox, s):
x1, y1, x2, y2 = bbox
mask = np.ones((y2 - y1, x2 - x1, 3), np.float32)
mask[random.randint(0, y2 - y1 - s):random.randint(0, y2 - y1), random.randint(0, x2 - x1 - s):random.randint(0, x2 - x1)] = 0
image[y1:y2, x1:x2] = image[y1:y2, x1:x2] * mask
return image
# 测试
image = cv2.imread('test.jpg')
bbox = [100, 100, 200, 200]
image_crop = crop(image, bbox)
image_translate, bbox_translate = translate(image, bbox, 50, 50)
image_brightness = brightness(image, 1.5)
image_noise = noise(image)
image_rotate, bbox_rotate = rotate(image, bbox, 30)
image_flip, bbox_flip = flip(image, bbox)
image_cutout = cutout(image, bbox, 50)
# 相关问题:
--相关问题--:
相关推荐
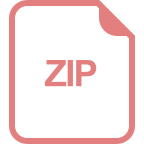
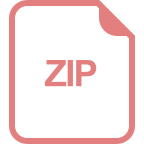
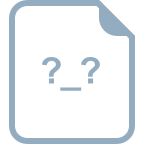
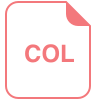
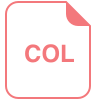
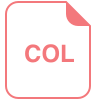
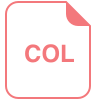
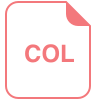









