帮我用python写一个五星红旗
时间: 2024-09-24 11:08:22 浏览: 56
在Python中,我们可以使用ASCII艺术来绘制简单的五星红旗。这里是一个基础的示例,展示如何打印一个竖直排列的五星红旗图案:
```python
def print_flying_flag():
# 旗面背景
flag_base = "*******************************************\n"
# 四颗小星
star_small = "* * * * *\n"
stars_small = star_small.replace(" ", "").replace("*", "#") * 4
# 一颗大星
star_large = "*********\n"
star_large = star_large.replace("*", "#")
# 组合并打印
flag = flag_base + "\n" + stars_small + "\n" + star_large
print(flag)
print_flying_flag()
```
这个脚本会输出一个简单的五星红旗形状,其中*代表白色部分,#代表红色部分。请注意这只是一个文字版的国旗,如果你需要更复杂的图形效果,可能需要借助第三方库如`PIL`来处理图像。
相关问题
帮我用python画一个五星红旗
好的,下面是用Python绘制五星红旗的代码:
```python
import turtle
# 设置窗口大小和背景色
turtle.setup(800, 600)
turtle.bgcolor('red')
# 画大五角星
turtle.penup()
turtle.goto(-180, 120)
turtle.pendown()
turtle.begin_fill()
turtle.color('yellow')
for i in range(5):
turtle.forward(120)
turtle.right(144)
turtle.end_fill()
# 画四个小五角星
turtle.penup()
turtle.goto(-60, 180)
turtle.pendown()
turtle.begin_fill()
for i in range(5):
turtle.forward(40)
turtle.right(144)
turtle.end_fill()
turtle.penup()
turtle.goto(-60, 90)
turtle.pendown()
turtle.begin_fill()
for i in range(5):
turtle.forward(40)
turtle.right(144)
turtle.end_fill()
turtle.penup()
turtle.goto(20, 150)
turtle.pendown()
turtle.begin_fill()
for i in range(5):
turtle.forward(40)
turtle.right(144)
turtle.end_fill()
turtle.penup()
turtle.goto(20, 60)
turtle.pendown()
turtle.begin_fill()
for i in range(5):
turtle.forward(40)
turtle.right(144)
turtle.end_fill()
# 隐藏画笔
turtle.hideturtle()
# 显示绘图窗口
turtle.done()
```
运行代码后,会弹出一个绘图窗口,显示出五星红旗的图案。
用python写一个五星红旗
今天是2020年5月25日,用python写一个五星红旗可以这样:
from turtle import *
penup()
goto(-200, 100)
pendown()
fillcolor("red")
begin_fill()
for _ in range(2):
forward(400)
right(90)
forward(300)
right(90)
end_fill()
# 画五颗星
penup()
goto(-180, 70)
pendown()
fillcolor("yellow")
begin_fill()
for _ in range(5):
forward(15)
right(144)
end_fill()
penup()
goto(-140, 70)
pendown()
fillcolor("yellow")
begin_fill()
for _ in range(5):
forward(15)
right(144)
end_fill()
penup()
goto(-100, 70)
pendown()
fillcolor("yellow")
begin_fill()
for _ in range(5):
forward(15)
right(144)
end_fill()
penup()
goto(-60, 70)
pendown()
fillcolor("yellow")
begin_fill()
for _ in range(5):
forward(15)
right(144)
end_fill()
penup()
goto(-20, 70)
pendown()
fillcolor("yellow")
begin_fill()
for _ in range(5):
forward(15)
right(144)
end_fill()
done()
阅读全文
相关推荐
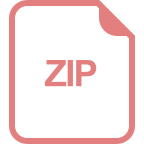
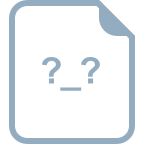













