#include <nlohmann/json.hpp>如何打印JSON格式
时间: 2023-12-29 16:02:41 浏览: 142
可以使用 nlohmann/json 库中的 `<<` 运算符将 JSON 格式的对象打印出来。示例代码如下:
```
#include <iostream>
#include <nlohmann/json.hpp>
using json = nlohmann::json;
int main() {
json my_json = {
{"name", "John"},
{"age", 30},
{"isStudent", true},
{"hobbies", {"reading", "swimming", "coding"}},
{"address", {
{"street", "Main Street"},
{"city", "New York"},
{"zip", "10001"}
}}
};
std::cout << my_json.dump() << std::endl;
return 0;
}
```
在上面的示例代码中,我们将 JSON 格式的对象 `my_json` 使用 `dump()` 方法转换为字符串,并使用 `std::cout` 输出到控制台。注意,`dump()` 方法默认使用缩进格式输出,可以通过传入参数来指定其他输出格式。
相关问题
#include <nlohmann/json.hpp>定义一个JSON格式的对象
可以使用 nlohmann::json 类来定义一个 JSON 格式的对象,示例代码如下:
```
#include <nlohmann/json.hpp>
using json = nlohmann::json;
int main() {
json my_json = {
{"name", "John"},
{"age", 30},
{"isStudent", true},
{"hobbies", {"reading", "swimming", "coding"}},
{"address", {
{"street", "Main Street"},
{"city", "New York"},
{"zip", "10001"}
}}
};
return 0;
}
```
在上面的示例代码中,我们定义了一个名为 `my_json` 的 JSON 格式的对象,并初始化了其中的一些属性。可以看到,nlohmann/json 库提供了非常方便的方式来定义和操作 JSON 格式的对象。
#include <nlohmann/json.hpp>把一个JSON格式的对象作为另一个JSON格式的成员
使用nlohmann/json库可以很方便地将一个JSON格式的对象作为另一个JSON格式的成员。以下是一个示例代码:
```cpp
#include <iostream>
#include <nlohmann/json.hpp>
using json = nlohmann::json;
int main() {
json person = {
{"name", "John"},
{"age", 30},
{"address", {
{"street", "123 Main St"},
{"city", "Anytown"},
{"state", "CA"},
{"zip", "12345"}
}}
};
std::cout << person.dump() << std::endl;
}
```
在此示例中,我们创建了一个名为"person"的JSON对象,其中包含"name"、"age"和"address"三个键。"address"键的值是另一个JSON对象,其中包含"street"、"city"、"state"和"zip"这四个键。
使用"dump()"函数将"person"对象转换为字符串输出,结果如下:
```
{
"address": {
"city": "Anytown",
"state": "CA",
"street": "123 Main St",
"zip": "12345"
},
"age": 30,
"name": "John"
}
```
阅读全文
相关推荐
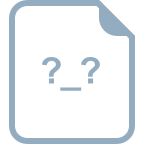
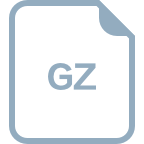
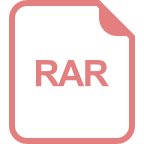













