用python写一个遍历文件夹及子文件夹中所有doc/docx文件的函数
时间: 2024-12-15 13:20:13 浏览: 25
在Python中,你可以使用`os`和`glob`模块来遍历文件夹及其子文件夹中的特定类型的文件,如`.doc`和`.docx`。这里有一个简单的函数示例:
```python
import os
import glob
def find_doc_files(start_path):
doc_files = []
for root, dirs, files in os.walk(start_path): # 使用os.walk遍历目录
for file in files:
if file.endswith(('.doc', '.docx')): # 检查文件是否为.doc或.docx
full_path = os.path.join(root, file) # 获取完整路径
doc_files.append(full_path)
return doc_files
# 调用函数,传入你想要搜索的根目录路径
files = find_doc_files('/path/to/start/directory')
```
这个函数会返回一个包含所有找到的`.doc`和`.docx`文件完整路径的列表。
相关问题
用python写一个遍历文件夹及子文件夹中所有doc/docx文件并且替换其中指定汉字的函数
在Python中,你可以使用`os`模块来遍历文件夹及其子文件夹,并结合`pathlib`库来处理文件路径,`openpyxl`或`python-docx`库来操作`.doc`或`.docx`文档。这里是一个基础的函数示例,它会查找并替换指定的汉字:
```python
import os
from typing import List
import docx
def replace_char_in_doc_files(root_folder: str, target_char: str, replacement_char: str) -> List[str]:
replaced_files = []
# 遍历文件夹及其子文件夹
for dirpath, _, filenames in os.walk(root_folder):
for filename in filenames:
if filename.endswith(('.doc', '.docx')):
file_path = os.path.join(dirpath, filename)
try:
# 打开文档
with docx.Document(file_path) as doc:
# 搜索并替换目标字符
for paragraph in doc.paragraphs:
text = paragraph.text
new_text = text.replace(target_char, replacement_char)
# 如果文本有变化,保存新的文档
if text != new_text:
new_file_name = f"{filename}_replaced_{target_char}_{replacement_char}"
new_file_path = os.path.join(dirpath, new_file_name)
doc.save(new_file_path)
replaced_files.append(new_file_path)
except Exception as e:
print(f"Error processing {file_path}: {e}")
return replaced_files
# 使用函数
root_folder = 'your_directory_path' # 替换为你的文件夹路径
target_char = '原字符' # 要替换的目标字符
replacement_char = '新字符' # 替换的新字符
replaced_files = replace_char_in_doc_files(root_folder, target_char, replacement_char)
用python输入当前路径遍历文件夹中和子文件夹的ts文件 转化为word文件
您好,我可以回答这个问题。您可以使用Python的os和docx库来实现这个功能。具体步骤如下:
1. 导入os和docx库
import os
from docx import Document
2. 定义一个函数,用于遍历文件夹中的ts文件并将其转化为word文件
def convert_ts_to_word(path):
for root, dirs, files in os.walk(path):
for file in files:
if file.endswith('.ts'):
# 读取ts文件内容
with open(os.path.join(root, file), 'r', encoding='utf-8') as f:
content = f.read()
# 创建word文档
doc = Document()
# 将ts文件内容写入word文档
doc.add_paragraph(content)
# 保存word文档
doc.save(os.path.join(root, file.replace('.ts', '.docx')))
3. 调用函数并传入当前路径
convert_ts_to_word(os.getcwd())
这样,当前路径下的所有ts文件都会被转化为word文件,并保存在相同的文件夹中。
阅读全文
相关推荐
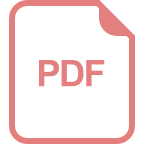
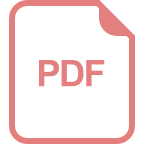
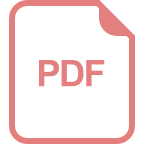













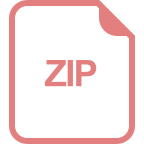