vue 鼠标悬停的位置 ctrl 滚轮放大 放大后可以拖拽
时间: 2023-10-01 13:03:17 浏览: 174
可以使用Vue和CSS来实现鼠标悬停放大和拖拽的效果,具体实现步骤如下:
1. 在Vue组件中设置放大的初始状态和样式,例如:
```html
<template>
<div class="container" :class="{ zoomed: zoomed }" @mousemove="handleMouseMove" @mouseleave="handleMouseLeave" @wheel="handleWheel">
<img src="./image.jpg" alt="image" :style="{ transform: `scale(${scale})`, left: `${left}px`, top: `${top}px` }">
</div>
</template>
<script>
export default {
data() {
return {
zoomed: false, // 初始状态为未放大
scale: 1, // 初始缩放比例为1
left: 0, // 初始位置为0
top: 0
}
},
methods: {
handleMouseMove(event) {
// 鼠标悬停时计算位置
if (this.zoomed) {
const containerRect = this.$el.getBoundingClientRect()
const x = event.clientX - containerRect.left
const y = event.clientY - containerRect.top
const centerX = containerRect.width / 2
const centerY = containerRect.height / 2
const moveX = (x - centerX) / centerX * 10
const moveY = (y - centerY) / centerY * 10
this.left = moveX
this.top = moveY
}
},
handleMouseLeave() {
// 鼠标离开时重置位置
if (this.zoomed) {
this.left = 0
this.top = 0
}
},
handleWheel(event) {
// 滚轮放大缩小
event.preventDefault()
if (event.ctrlKey) {
const scale = this.scale - event.deltaY / 1000
this.scale = Math.max(0.1, Math.min(scale, 3)) // 缩放比例限制在0.1~3之间
this.zoomed = this.scale > 1 // 根据缩放比例判断是否放大
}
}
}
}
</script>
<style>
.container {
position: relative;
width: 500px;
height: 500px;
overflow: hidden;
cursor: zoom-in;
transition: transform 0.3s, width 0.3s, height 0.3s;
}
.container.zoomed {
cursor: move;
}
.container img {
position: absolute;
width: 100%;
height: 100%;
object-fit: cover;
transition: transform 0.3s, left 0.3s, top 0.3s;
}
</style>
```
2. 在CSS中设置鼠标悬停和拖拽的样式,例如:
```css
.container:hover {
cursor: zoom-out;
}
.container.zoomed {
cursor: move;
}
.container.zoomed img {
cursor: move;
}
```
这样就可以实现鼠标悬停放大和拖拽的效果了。注意,为了避免缩放过大或过小导致页面布局错乱,可以对缩放比例进行限制。同时,为了方便拖拽,可以在放大状态下设置鼠标为move样式。
阅读全文
相关推荐


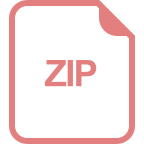
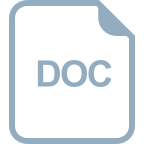
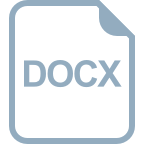
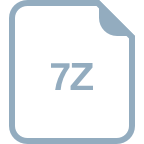
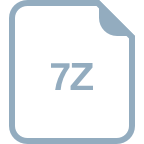
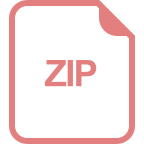
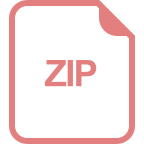
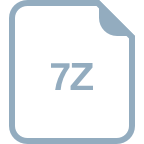