html如何实现动态单页滚动
时间: 2023-07-07 10:06:54 浏览: 145
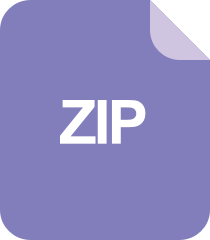
单页滚动HTML5模板
HTML本身无法实现动态单页滚动,但可以通过JavaScript和CSS来实现。
通常情况下,实现动态单页滚动需要用到以下步骤:
1. 创建一个包含所有内容的单页应用程序,并将所有内容放在同一个HTML文件中。
2. 使用CSS设置整个页面的高度为视口高度,以便在滚动时整个页面都能够滚动。
3. 使用JavaScript设置事件监听器,以便在单击导航链接时滚动到相应的部分。
4. 在导航链接中使用锚点(#)来标识每个部分的位置。
下面是一个简单的示例代码,演示如何使用JavaScript和CSS实现动态单页滚动:
HTML代码:
```
<!DOCTYPE html>
<html>
<head>
<title>动态单页滚动</title>
<style>
body {
margin: 0;
padding: 0;
height: 100vh;
scroll-behavior: smooth; /* 实现平滑滚动 */
}
section {
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
font-size: 5rem;
}
#section1 {
background-color: red;
}
#section2 {
background-color: blue;
}
#section3 {
background-color: green;
}
#section4 {
background-color: yellow;
}
nav {
position: fixed;
top: 0;
left: 0;
width: 100%;
background-color: rgba(255, 255, 255, 0.8);
display: flex;
justify-content: space-around;
align-items: center;
padding: 1rem;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3);
}
nav a {
text-decoration: none;
color: black;
font-size: 2rem;
transition: all 0.3s ease-in-out;
}
nav a:hover {
transform: scale(1.2);
}
</style>
</head>
<body>
<nav>
<a href="#section1">Section 1</a>
<a href="#section2">Section 2</a>
<a href="#section3">Section 3</a>
<a href="#section4">Section 4</a>
</nav>
<section id="section1">Section 1</section>
<section id="section2">Section 2</section>
<section id="section3">Section 3</section>
<section id="section4">Section 4</section>
<script>
// 获取所有的导航链接
const links = document.querySelectorAll('nav a');
// 为每个链接添加事件监听器
links.forEach(link => {
link.addEventListener('click', e => {
// 阻止默认行为
e.preventDefault();
// 获取目标元素的ID
const targetId = link.getAttribute('href');
// 滚动到目标元素
document.querySelector(targetId).scrollIntoView({
behavior: 'smooth'
});
});
});
</script>
</body>
</html>
```
在上面的示例代码中,使用了CSS的`height: 100vh`将整个页面的高度设置为视口高度,以便在滚动时整个页面都能够滚动。同时,使用锚点(#)来标识每个部分的位置,并在导航链接中使用锚点。在JavaScript中,使用事件监听器来监听导航链接的单击事件,并使用`scrollIntoView()`方法滚动到相应的部分。
阅读全文
相关推荐
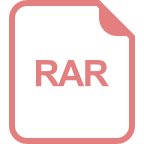
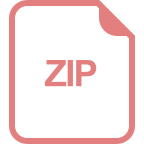
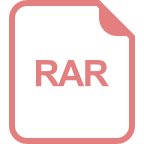
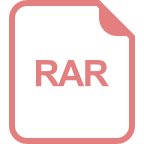
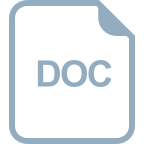
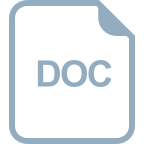

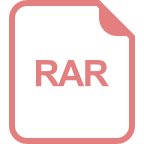
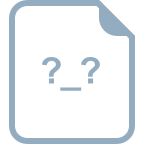
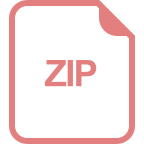
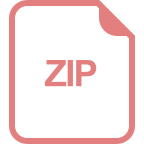
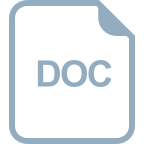
