写一段简短的代码在idea上用javafx开发五子棋
时间: 2024-12-21 12:24:06 浏览: 13
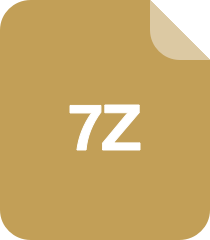
在IntelliJ IDEA 中使用 Gradle JavaFx
在IntelliJ IDEA上用JavaFX开发五子棋游戏的一个基本框架可能会包含一些核心组件和逻辑。以下是一个简单的示例,展示如何开始:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class GoGame extends Application {
private Circle[][] board = new Circle[15][15]; // 15x15的游戏棋盘
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("五子棋");
StackPane root = new StackPane();
Scene scene = new Scene(root, 400, 400);
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
board[i][j] = new Circle(30);
if (i % 2 == 0 || j % 2 == 0) { // 简单地将黑色格子放在偶数行、列
board[i][j].setFill(Color.BLACK);
} else {
board[i][j].setFill(Color.WHITE);
}
board[i][j].setTranslateX(j * 40);
board[i][j].setTranslateY(i * 40);
root.getChildren().add(board[i][j]);
}
}
Button currentPlayerButton = new Button("当前玩家");
currentPlayerButton.setOnAction(event -> changePlayer()); // 按钮切换玩家
root.getChildren().add(currentPlayerButton);
primaryStage.setScene(scene);
primaryStage.show();
// 更复杂的逻辑会涉及事件监听、点击处理和AI算法等
// 这里仅作为基础布局示例
}
private void changePlayer() {
// 实现玩家切换逻辑,这里省略
}
public static void main(String[] args) {
launch(args);
}
}
```
这只是一个非常基础的版本,实际应用中需要添加更多功能如用户交互、检测胜利条件、AI对手等。请注意,这个代码片段没有处理游戏循环和逻辑,这些都是五子棋游戏的核心部分。
阅读全文
相关推荐
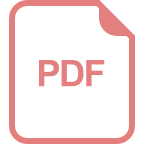
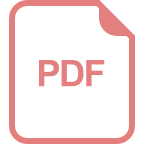
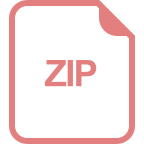
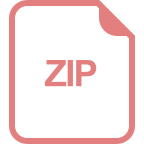
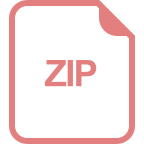
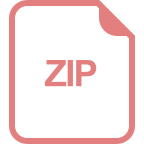





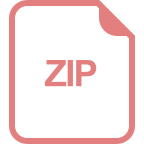


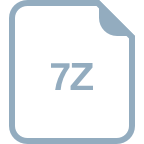