模拟退火的一刀切板材排版C#代码
时间: 2023-11-14 21:07:38 浏览: 130
以下是使用模拟退火算法的一刀切板材排版的 C# 代码示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
namespace SimulatedAnnealing
{
class Program
{
static void Main(string[] args)
{
// 定义板材大小和零件列表
int boardWidth = 100;
int boardHeight = 50;
List<Part> parts = new List<Part>()
{
new Part("Part1", 20, 20),
new Part("Part2", 30, 10),
new Part("Part3", 10, 30),
new Part("Part4", 40, 20),
new Part("Part5", 20, 30)
};
// 初始化排版方案
Layout currentLayout = new Layout(boardWidth, boardHeight, parts);
// 计算初始能量(即面积利用率)
double currentEnergy = currentLayout.CalculateEnergy();
// 定义模拟退火相关参数
double initialTemperature = 1000;
double finalTemperature = 1;
double coolingRatio = 0.95;
int maxIterations = 10000;
// 执行模拟退火算法
Random random = new Random();
double temperature = initialTemperature;
for (int i = 0; i < maxIterations && temperature > finalTemperature; i++)
{
// 随机生成新的排版方案
Layout newLayout = currentLayout.GenerateNeighbor(random);
// 计算新方案的能量
double newEnergy = newLayout.CalculateEnergy();
// 判断是否接受新方案
double deltaEnergy = newEnergy - currentEnergy;
if (deltaEnergy < 0 || Math.Exp(-deltaEnergy / temperature) > random.NextDouble())
{
currentLayout = newLayout;
currentEnergy = newEnergy;
}
// 降低温度
temperature *= coolingRatio;
}
// 输出最终排版方案和能量
Console.WriteLine(currentLayout);
Console.WriteLine("Final energy: " + currentEnergy);
}
}
// 零件类
class Part
{
public string Name { get; set; }
public int Width { get; set; }
public int Height { get; set; }
public Part(string name, int width, int height)
{
Name = name;
Width = width;
Height = height;
}
}
// 排版方案类
class Layout
{
public int BoardWidth { get; set; }
public int BoardHeight { get; set; }
public List<Part> Parts { get; set; }
public Layout(int boardWidth, int boardHeight, List<Part> parts)
{
BoardWidth = boardWidth;
BoardHeight = boardHeight;
Parts = parts;
}
// 随机生成邻居方案
public Layout GenerateNeighbor(Random random)
{
Layout newLayout = new Layout(BoardWidth, BoardHeight, new List<Part>(Parts));
int index = random.Next(newLayout.Parts.Count);
Part part = newLayout.Parts[index];
part.Width = random.Next(BoardWidth - part.Width + 1);
part.Height = random.Next(BoardHeight - part.Height + 1);
return newLayout;
}
// 计算面积利用率
public double CalculateEnergy()
{
int totalArea = BoardWidth * BoardHeight;
int usedArea = Parts.Sum(part => part.Width * part.Height);
return (double)usedArea / totalArea;
}
// 输出排版方案
public override string ToString()
{
string result = "Layout:\n";
foreach (Part part in Parts)
{
result += part.Name + ": (" + part.Width + ", " + part.Height + ")\n";
}
return result;
}
}
}
```
在此示例中,我们定义了一个 `Part` 类来表示零件,包括名称、宽度和高度;定义了一个 `Layout` 类来表示排版方案,包括板材大小和零件列表,并提供了生成邻居方案、计算能量(面积利用率)和输出排版方案的方法。然后,我们使用模拟退火算法来搜索最优排版方案。在算法执行过程中,我们随机生成新的排版方案,并计算其能量。根据模拟退火的原理,我们以一定的概率接受新方案,以便跳出局部最优解。最后,我们输出最终的排版方案和能量。
阅读全文
相关推荐
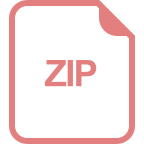
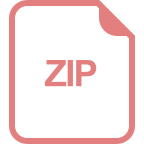
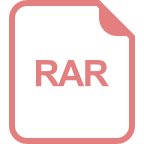
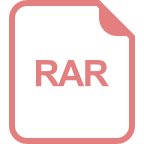
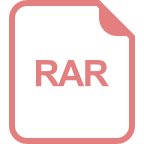
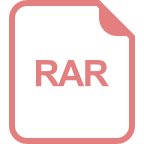
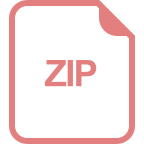
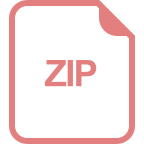
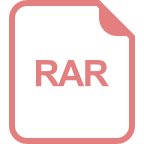
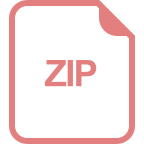
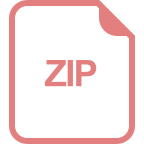
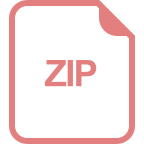
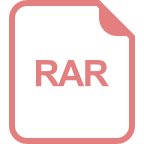