用C++实现在给定元素e最后一次出现的位置后插入新元素x,若表中不存在元素e则将x插入到表尾,返回值true/false表示操作是否成功:bool ListInsert(SqList &L,int i,ElemType e)
时间: 2024-10-25 10:14:18 浏览: 20
在C++中,你可以通过遍历链表的方式来实现这个功能。首先,我们需要定义一个`SqList`结构体或类,它通常包含一个指向链表头节点的指针。以下是简单的步骤:
```cpp
class SqList {
public:
// 假设Node是一个链表节点结构,包含数据成员val和next指针
struct Node {
ElemType val;
Node* next;
};
// 初始化、获取头节点等其他必要的成员函数
private:
Node* head; // 链表头指针
};
// 实现插入元素的操作
bool ListInsert(SqList& L, int i, const ElemType& e) {
bool found = false; // 标记是否找到e
Node* current = L.head;
Node* newNode = new Node(); // 创建新节点
newNode->val = x; // 将x赋值给新节点
if (i == 0) { // 如果索引为0,直接插入到头部
newNode->next = L.head;
L.head = newNode;
found = true;
} else {
for (int index = 0; current != nullptr && index < i; ++index, current = current->next) {
if (current->val == e) { // 找到e
newNode->next = current->next;
current->next = newNode;
found = true;
break;
}
}
if (!found) { // 没有找到e,插入到链表尾部
newNode->next = nullptr;
if (L.head == nullptr) { // 如果链表为空,直接插入
L.head = newNode;
} else {
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
}
return found; // 返回是否找到并插入了e
}
```
阅读全文
相关推荐
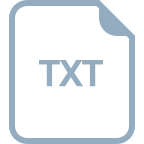
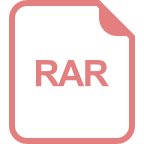
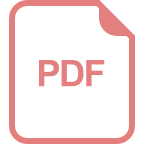















