python base64照片转blob
时间: 2023-10-05 15:08:11 浏览: 173
可以使用以下代码将 Python 中的 base64 照片转换为 blob:
```python
import base64
# 读取 base64 照片
with open("photo.txt", "r") as f:
photo_base64 = f.read()
# 将 base64 照片转换为二进制数据
photo_blob = base64.b64decode(photo_base64)
# 将二进制数据写入文件
with open("photo.jpg", "wb") as f:
f.write(photo_blob)
```
以上代码将 base64 照片从文件 "photo.txt" 中读取,将其转换为二进制数据,并将其写入文件 "photo.jpg" 中。你可以根据需要修改文件名和路径。
相关问题
python将bolb格式转成base64
在Python中,将blob(二进制大对象)转换为Base64编码通常是为了在网络传输或者存储文本形式的数据。你可以使用内置的`base64`模块来完成这个操作。下面是一个简单的例子:
```python
import base64
# 假设你有一个blob数据
binary_data = b"your_binary_data_here"
# 将blob转换为bytes
encoded_data = binary_data
# 使用base64编码
encoded_string = base64.b64encode(encoded_data)
# 如果需要字符串形式,可以解码为str(去除前导`b`)
encoded_string_str = encoded_string.decode('utf-8')
print(f"Base64编码后的字符串: {encoded_string_str}")
# 如果需要原始格式的Base64字符串(包含前缀`data:`),可以这样处理
base64_bytes_with_prefix = "data:image/png;base64," + encoded_string_str
```
如果你的blob数据是从文件读取或者网络请求得到的,记得先将其读入bytes。
图片BASE64加密保存到数据库Blob类型中(放入数据库,并取出生成图片)
图片BASE64编码是一种将图像数据转换为纯文本格式的方式,方便在网络上传输或存储,尤其是当直接保存原始二进制数据(如JPEG、PNG等)会超出字符集限制时。将图片BASE64编码后保存到数据库的BLOB(Binary Large Object)字段中,可以有效地存储非结构化的二进制数据。
以下是基本步骤:
1. **读取图片并编码**:使用编程语言(如Python、Java等),从文件系统加载图片,然后使用内置库将其转换为Base64字符串。
```python
import base64
with open('image.jpg', 'rb') as img_file:
image_data = img_file.read()
encoded_image = base64.b64encode(image_data).decode('utf-8')
```
2. **插入到数据库**:将Base64编码后的字符串作为值,插入到Blob类型的列中。例如,在SQL中可能是这样的:
```sql
INSERT INTO images (image_data) VALUES ('data:image/jpeg;base64,' || :encoded_image);
```
这里`:encoded_image`是一个占位符,实际操作时需要替换为实际的Base64字符串。
3. **从数据库取出并解码**:当你想要获取图片时,从数据库查询出来Base64编码的字符串,然后反序列化成字节流:
```python
decoded_image = base64.b64decode(encoded_image)
```
最后,你可以创建一个新的文件并将字节流写入其中,或者直接显示为图片。
阅读全文
相关推荐
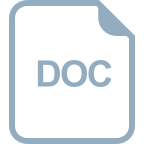
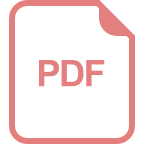
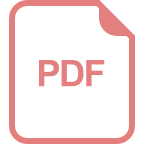
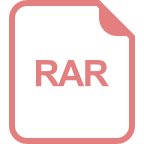
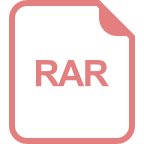
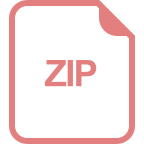
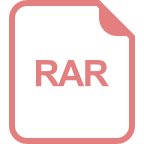
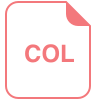
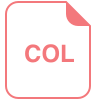
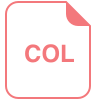
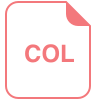


