用Kotlin写一个为WebView添加操作栏的代码
时间: 2024-05-07 11:21:48 浏览: 169
以下是一个为WebView添加操作栏的 Kotlin 代码示例:
首先,在布局文件中添加一个 WebView 和一个操作栏布局:
```
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<WebView
android:id="@+id/web_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<LinearLayout
android:id="@+id/toolbar_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_alignParentTop="true"
android:background="#FFFFFF"
android:elevation="4dp">
<ImageButton
android:id="@+id/back_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_arrow_back"
android:padding="10dp" />
<ImageButton
android:id="@+id/forward_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_arrow_forward"
android:padding="10dp" />
<ImageButton
android:id="@+id/refresh_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_refresh"
android:padding="10dp" />
</LinearLayout>
</RelativeLayout>
```
然后,在 Kotlin 代码中,找到 WebView 和操作栏布局:
```
val webView = findViewById<WebView>(R.id.web_view)
val toolbarLayout = findViewById<LinearLayout>(R.id.toolbar_layout)
```
接下来,给操作栏按钮添加点击事件和实现相应的 WebView 方法:
```
val backButton = findViewById<ImageButton>(R.id.back_button)
backButton.setOnClickListener {
if (webView.canGoBack()) {
webView.goBack()
}
}
val forwardButton = findViewById<ImageButton>(R.id.forward_button)
forwardButton.setOnClickListener {
if (webView.canGoForward()) {
webView.goForward()
}
}
val refreshButton = findViewById<ImageButton>(R.id.refresh_button)
refreshButton.setOnClickListener {
webView.reload()
}
```
最后,设置 WebView 的客户端,以便在页面加载时更新操作栏按钮状态和标题:
```
webView.webChromeClient = object : WebChromeClient() {
override fun onProgressChanged(view: WebView?, newProgress: Int) {
// 当页面加载时更新操作栏按钮状态
backButton.isEnabled = webView.canGoBack()
forwardButton.isEnabled = webView.canGoForward()
}
override fun onReceivedTitle(view: WebView?, title: String?) {
// 当页面标题变化时更新操作栏标题
supportActionBar?.title = title
}
}
```
以上就是一个简单的为 WebView 添加操作栏的 Kotlin 代码示例。
阅读全文
相关推荐
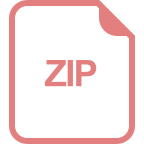
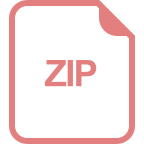
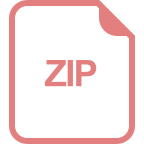





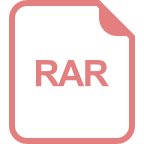
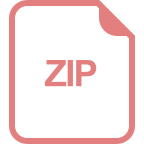
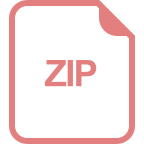
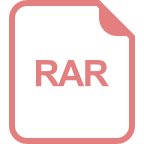
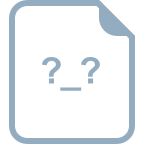
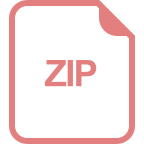
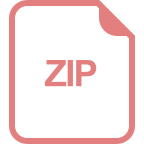
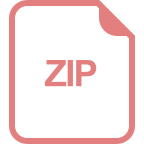
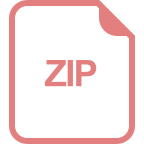
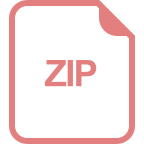
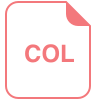