c++ 面向对象 小游戏
时间: 2024-04-28 07:17:04 浏览: 253
C++面向对象小游戏是一种基于C++面向对象编程思想的游戏开发方式。在这种游戏中,所有的游戏元素都被抽象成对象,每个对象都有自己的属性和方法。这种开发方式可以使游戏的开发更加模块化和可维护,同时也可以提高游戏的性能和可扩展性。
在这个引用中,ChessBoard.h是一个棋盘类的头文件,它定义了一个棋盘类,这个类包含了一个15*15的字符数组,用于表示棋盘上的棋子。这个类还包含了一些方法,用于在棋盘上放置棋子、判断胜负等操作。
如果你想开发一个C++面向对象小游戏,你需要先确定游戏的类型和规则,然后设计游戏的各个元素,例如游戏场景、角色、道具等。接着,你需要使用C++面向对象编程的思想,将这些元素抽象成对象,并定义它们的属性和方法。最后,你需要将这些对象组合起来,实现游戏的逻辑和交互。
阅读全文
相关推荐
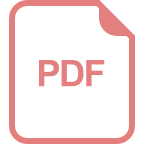
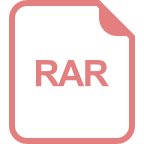
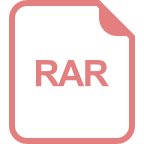
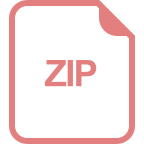
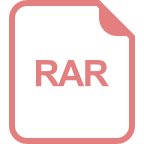
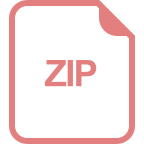
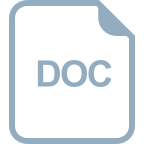
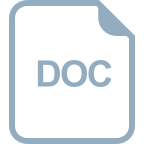
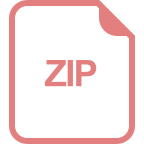
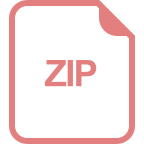
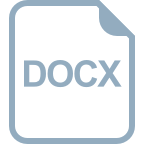
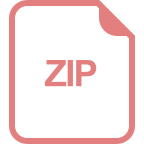
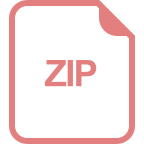
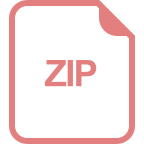
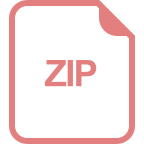
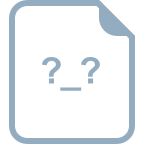