如何使用js和css和html绘制出一个带有动画的冰墩墩
时间: 2024-05-12 10:21:07 浏览: 88
以下是一个使用HTML、CSS和JavaScript绘制带有动画的冰墩墩的示例:
HTML代码:
```html
<div class="ice-container">
<div class="ice-block"></div>
<div class="ice-block"></div>
<div class="ice-block"></div>
<div class="ice-block"></div>
<div class="ice-block"></div>
<div class="ice-block"></div>
<div class="ice-block"></div>
<div class="ice-block"></div>
</div>
```
CSS代码:
```css
.ice-container {
position: relative;
width: 200px;
height: 400px;
margin: 50px auto;
perspective: 1000px;
}
.ice-block {
position: absolute;
width: 100px;
height: 100px;
background-color: #e0ffff;
border-radius: 50%;
transform-style: preserve-3d;
animation: spin 10s linear infinite;
}
.ice-block:nth-child(1) {
top: 0;
left: 50px;
transform: rotateX(90deg) translateZ(50px);
}
.ice-block:nth-child(2) {
top: 50px;
left: 0;
transform: rotateY(90deg) translateZ(50px);
}
.ice-block:nth-child(3) {
top: 100px;
left: 50px;
transform: rotateX(-90deg) translateZ(50px);
}
.ice-block:nth-child(4) {
top: 50px;
left: 100px;
transform: rotateY(-90deg) translateZ(50px);
}
.ice-block:nth-child(5) {
top: 50px;
left: 50px;
transform: translateZ(150px);
}
.ice-block:nth-child(6) {
top: 150px;
left: 50px;
transform: rotateX(-90deg) translateZ(250px);
}
.ice-block:nth-child(7) {
top: 150px;
left: 50px;
transform: translateZ(350px);
}
.ice-block:nth-child(8) {
top: 250px;
left: 50px;
transform: rotateX(-90deg) translateZ(450px);
}
@keyframes spin {
from {
transform: rotateY(0);
}
to {
transform: rotateY(360deg);
}
}
```
JavaScript代码:
```javascript
// 为冰墩墩添加鼠标悬停事件
document.querySelector('.ice-container').addEventListener('mouseover', function() {
// 将所有冰块的背景色变为白色
document.querySelectorAll('.ice-block').forEach(function(block) {
block.style.backgroundColor = '#fff';
});
});
// 为冰墩墩添加鼠标离开事件
document.querySelector('.ice-container').addEventListener('mouseout', function() {
// 将所有冰块的背景色变回原来的颜色
document.querySelectorAll('.ice-block').forEach(function(block, index) {
block.style.backgroundColor = '#e0ffff';
// 为每个冰块设置不同的延迟时间,使它们逐个变回原来的颜色
setTimeout(function() {
block.style.transition = 'background-color 0.5s ease';
}, index * 100);
});
});
```
这段代码定义了一个.container元素,其中包含八个.ice-block元素,每个元素表示冰墩墩的一个面。通过CSS的transform属性和animation属性,可以使这些元素旋转并形成3D效果。同时,JavaScript代码为这个冰墩墩添加了鼠标悬停和离开事件,使其可以响应用户的交互。
相关推荐
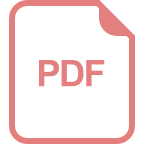











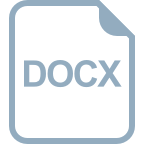
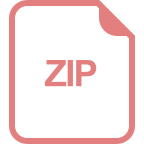
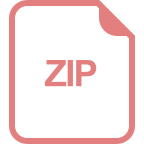
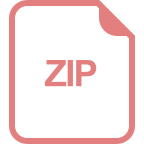
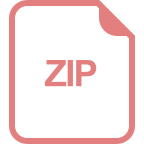