编写一个C#程序。保证输入的全是十六进制情况下,输入一个完整字节后若用户继续输入,则在这个完整字节后面加一个空格并显示在富文本框中,光标要保证在最后。如何输入完整字节后面没有再输入内容则不在这个完整 字节后面加空格。
时间: 2024-10-25 07:11:45 浏览: 17
在C#中,你可以使用`Console.ReadLine()`读取用户的输入,并检查其是否为有效的十六进制字符。如果用户输入的是完整的字节,然后加上空格并在富文本框中显示。这里是一个简单的示例:
```csharp
using System;
using System.Windows.Forms; // 引入用于富文本框的命名空间
public partial class MainForm : Form {
private TextBox textBoxOutput;
public MainForm() {
InitializeComponent();
textBoxOutput = new TextBox { Location = new Point(10, 10), Size = new Size(100, 20) };
Controls.Add(textBoxOutput);
}
private void ReadInput() {
string input = Console.ReadLine();
// 检查输入是否是有效的16进制字符,如果是,则处理它
if (IsValidHex(input)) {
byte byteValue;
if (byte.TryParse(input, out byteValue)) {
string formattedValue = $"{input} "; // 添加空格
textBoxOutput.Text += formattedValue;
textBoxOutput.Select(textBoxOutput.Text.Length, 0); // 设置光标到末尾
}
}
}
private bool IsValidHex(string hex) {
foreach (char c in hex) {
if (!Char.IsLetterOrDigit(c) && c != 'x' && c != 'X') {
return false;
}
}
return true;
}
static void Main() {
Application.Run(new MainForm());
}
// 程序运行时持续读取输入
private void ConsoleAppLoop() {
while (true) {
ReadInput();
}
}
}
// 注意:在实际应用中,你可能需要考虑将这部分放到后台线程,以避免阻塞UI线程
```
在此代码中,`ReadInput`函数会一直循环等待用户输入。当用户停止输入时,程序会继续运行而不会暂停。如果你想在控制台应用程序中看到实时更新,可以添加`ConsoleAppLoop`函数。
阅读全文
相关推荐
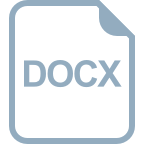
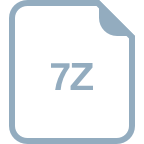
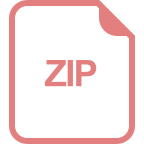
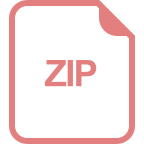
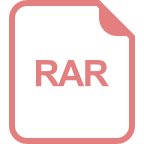
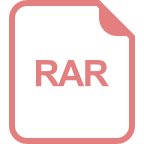
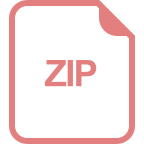
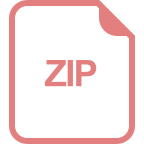
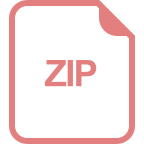
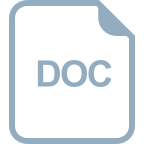
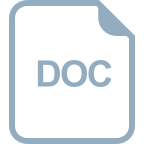
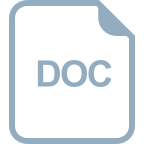
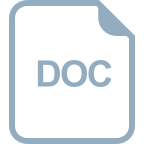
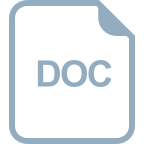




