(Geometry: intersecting point) Suppose two line segments intersect. The two end-points for the first line segment are (x1, y1) and (x2, y2) and for the second line segment are (x3, y3) and (x4, y4).Write a program that prompts the user to enter these four endpoints and displays the intersecting point. the intersecting point can be found by solving a linear equation. Write the LinearEquation class in Programming to solve this equation. input style : Enter eight double number output style: Displays the intersecting point or The two lines are parallel
时间: 2024-03-10 09:45:44 浏览: 17
Here's one possible solution in Java:
```java
import java.util.Scanner;
public class IntersectingPoint {
public static void main(String[] args) {
// Prompt the user to enter the four endpoints
Scanner input = new Scanner(System.in);
System.out.print("Enter x1, y1, x2, y2, x3, y3, x4, y4: ");
double x1 = input.nextDouble();
double y1 = input.nextDouble();
double x2 = input.nextDouble();
double y2 = input.nextDouble();
double x3 = input.nextDouble();
double y3 = input.nextDouble();
double x4 = input.nextDouble();
double y4 = input.nextDouble();
// Create two LinearEquation objects for the two line segments
LinearEquation eq1 = new LinearEquation(y1 - y2, x2 - x1, y1*x2 - y2*x1);
LinearEquation eq2 = new LinearEquation(y3 - y4, x4 - x3, y3*x4 - y4*x3);
// Check if the two lines are parallel
if (eq1.isParallel(eq2)) {
System.out.println("The two lines are parallel");
} else {
// Compute the intersecting point
double x = eq1.getX(eq2);
double y = eq1.getY(eq2);
System.out.printf("The intersecting point is (%.2f, %.2f)\n", x, y);
}
}
}
class LinearEquation {
private double a, b, c;
public LinearEquation(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
public double getA() {
return a;
}
public double getB() {
return b;
}
public double getC() {
return c;
}
public boolean isParallel(LinearEquation other) {
return Math.abs(a * other.b - b * other.a) < 1e-6;
}
public double getX(LinearEquation other) {
return (c * other.b - b * other.c) / (a * other.b - b * other.a);
}
public double getY(LinearEquation other) {
return (a * other.c - c * other.a) / (a * other.b - b * other.a);
}
}
```
The program first prompts the user to enter the four endpoints, then creates two `LinearEquation` objects for the two line segments, and finally checks if the two lines are parallel or computes the intersecting point using the `getX` and `getY` methods of the `LinearEquation` class. The `isParallel` method checks if the two lines are parallel by comparing the slopes of the two lines, and the `getX` and `getY` methods solve the system of two linear equations for the intersecting point.
相关推荐
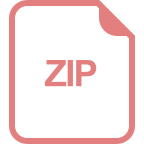
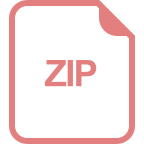
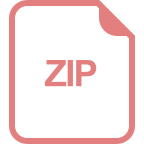














