写一段python基于yolov5框架人脸识别的代码
时间: 2023-05-26 16:03:35 浏览: 106
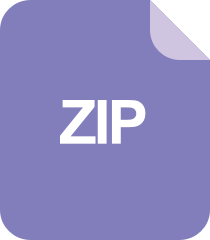
用YOLOV5实现人脸检测的代码,配置好系统可直接运行
实现基于yolov5框架的人脸识别代码如下:
```
import cv2
import numpy as np
import torch
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.torch_utils import select_device
# 加载模型
model = attempt_load("yolov5s.pt", map_location='cpu')
# 设置设备
device = select_device("cpu")
# 设置阈值
confidence = 0.4
iou_thresh = 0.5
# 加载类别名称
classes = ["face"]
# 开启摄像头
cap = cv2.VideoCapture(0)
# 循环读取帧
while True:
# 读取帧
ret, frame = cap.read()
if not ret:
break
# 转化为RGB
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 处理大小
img = cv2.resize(frame, (640, 640))
# 转化为张量
img = torch.from_numpy(img).to(device)
# 添加维度
img = img.permute(2, 0, 1).unsqueeze(0)
# 模型推理
with torch.no_grad():
output = model(img.float(), augment=False)[0]
# 处理坐标
boxes = output[..., :4]
boxes = scale_coords(img.shape[2:], boxes, frame.shape).round()
# 处理置信度和类别
confidence_scores = output[..., 4]
class_indexes = output[..., 5].long()
# 多类别处理
detections = []
for class_index in range(output.shape[2]-5):
class_mask = class_indexes == class_index
if not class_mask.any():
continue
class_boxes = boxes[class_mask]
class_confidence_scores = confidence_scores[class_mask]
# 多类别非极大抑制
class_detections = non_max_suppression(
torch.cat((class_boxes, class_confidence_scores.unsqueeze(1)), dim=1),
conf_thres=confidence,
iou_thres=iou_thresh,
multi_label=False,
classes=None,
agnostic=True
)
for detection in class_detections:
detection = detection.cpu().numpy()
detection = detection[0:4].astype(np.int)
detections.append(detection)
# 画人脸框
for box in detections:
x_min, y_min, x_max, y_max = box
cv2.rectangle(frame, (x_min, y_min), (x_max, y_max), (0, 255, 0), 2)
# 显示结果
cv2.imshow("face detection", frame)
# 退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头
cap.release()
# 关闭窗口
cv2.destroyAllWindows()
```
该代码首先加载yolov5模型,然后设置设备、阈值和类别名称。接下来开启摄像头,循环读取每一帧,并进行模型推理。模型输出包含置信度、类别和坐标信息,需要对其进行处理,得到每一个人脸框的坐标。最后,将每一个人脸框用矩形框住,并显示结果。
阅读全文
相关推荐
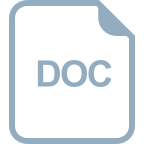
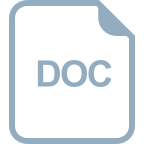
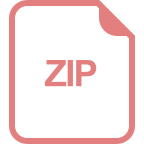
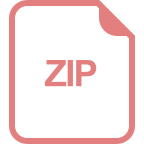
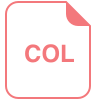

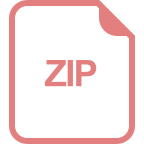
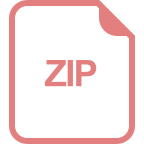
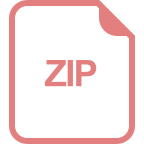
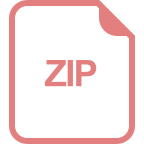
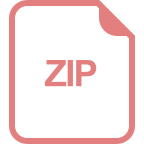
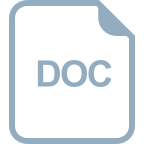
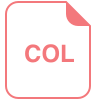
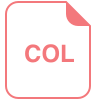
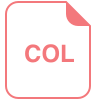
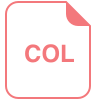
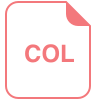
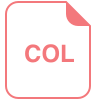