c#实现购物车前端和后端代码
时间: 2023-10-16 11:06:02 浏览: 233
这里是一个简单的 C# 购物车的前端和后端代码示例:
后端代码(使用 ASP.NET MVC):
```csharp
using System.Collections.Generic;
using System.Linq;
using System.Web.Mvc;
namespace ShoppingCart.Controllers
{
public class HomeController : Controller
{
// 商品列表
private static readonly List<Product> Products = new List<Product>
{
new Product {Id = 1, Name = "商品1", Price = 10},
new Product {Id = 2, Name = "商品2", Price = 20},
new Product {Id = 3, Name = "商品3", Price = 30},
new Product {Id = 4, Name = "商品4", Price = 40},
new Product {Id = 5, Name = "商品5", Price = 50},
};
// 购物车
private static readonly List<CartItem> CartItems = new List<CartItem>();
public ActionResult Index()
{
// 把商品列表传递给前端页面
ViewBag.Products = Products;
return View();
}
// 添加商品到购物车
public ActionResult AddToCart(int id)
{
var product = Products.FirstOrDefault(p => p.Id == id);
if (product != null)
{
// 查找购物车里是否已经有该商品,有则数量加 1,否则添加一条新的购物车项
var cartItem = CartItems.FirstOrDefault(ci => ci.Product.Id == product.Id);
if (cartItem != null)
{
cartItem.Quantity++;
}
else
{
CartItems.Add(new CartItem {Product = product, Quantity = 1});
}
}
return RedirectToAction("Index");
}
// 清空购物车
public ActionResult ClearCart()
{
CartItems.Clear();
return RedirectToAction("Index");
}
}
// 商品类
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
// 购物车项类
public class CartItem
{
public Product Product { get; set; }
public int Quantity { get; set; }
}
}
```
前端代码:
```html
@{
ViewBag.Title = "购物车";
}
<h2>商品列表</h2>
<ul>
@foreach (var product in ViewBag.Products)
{
<li>@product.Name - ¥@product.Price <a href="@Url.Action("AddToCart", new {id = product.Id})">加入购物车</a></li>
}
</ul>
<h2>购物车</h2>
@if (HomeController.CartItems.Count == 0)
{
<p>购物车是空的</p>
}
else
{
<ul>
@foreach (var cartItem in HomeController.CartItems)
{
<li>@cartItem.Product.Name - ¥@cartItem.Product.Price - 数量:@cartItem.Quantity</li>
}
</ul>
<p><a href="@Url.Action("ClearCart")">清空购物车</a></p>
}
```
这里的代码实现了一个简单的购物车功能,包括商品列表、添加商品到购物车、清空购物车等功能。当用户点击“加入购物车”按钮时,会将商品添加到后端的购物车列表中;当用户查看购物车时,会从后端获取购物车列表并显示在前端页面上。
阅读全文
相关推荐
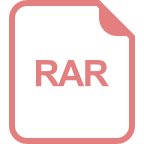
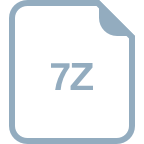
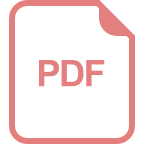
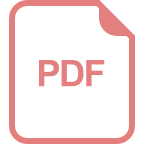
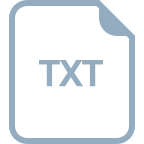
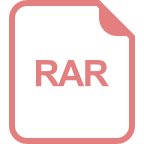
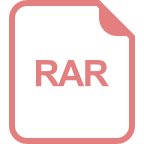
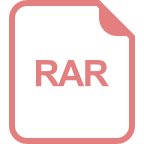
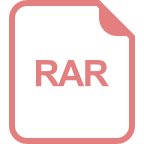
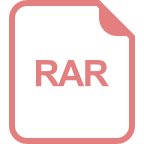
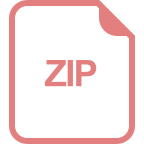
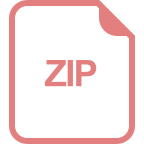
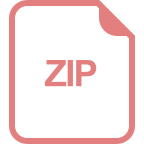
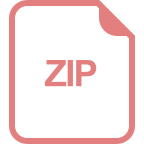
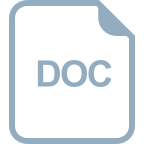
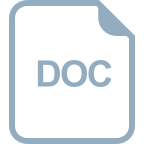
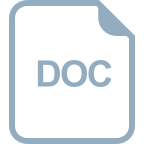