boost python 参数结构体指针_Boost-Python原始指针构造函数
时间: 2023-10-07 17:08:32 浏览: 159
好的,您的问题是关于 Boost Python 中如何处理参数为结构体指针的情况,以及如何使用 Boost Python 的原始指针构造函数。
首先,对于结构体指针参数,您可以使用 Boost Python 的 `boost::python::ptr` 类型来传递指针。例如,假设您有一个名为 `MyStruct` 的结构体,您可以在 Python 中创建一个 `MyStruct` 的实例,并将其传递给 C++ 函数,代码如下:
```c++
#include <boost/python.hpp>
struct MyStruct {
int x;
int y;
};
void my_func(boost::python::ptr<MyStruct> ptr) {
if (ptr.get()) {
std::cout << "x: " << ptr->x << ", y: " << ptr->y << std::endl;
} else {
std::cout << "ptr is null" << std::endl;
}
}
BOOST_PYTHON_MODULE(my_module) {
boost::python::class_<MyStruct>("MyStruct", boost::python::init<>())
.def_readwrite("x", &MyStruct::x)
.def_readwrite("y", &MyStruct::y);
boost::python::def("my_func", my_func);
}
```
然后在 Python 中创建一个 `MyStruct` 实例,并将其传递给 `my_func` 函数:
```python
import my_module
s = my_module.MyStruct()
s.x = 10
s.y = 20
my_module.my_func(s)
```
对于原始指针构造函数,您可以使用 `boost::python::make_constructor` 函数来创建一个构造函数,该构造函数接受一个原始指针作为参数。例如,假设您有一个名为 `MyClass` 的类,它有一个接受 `int` 和 `double` 参数的构造函数,并且您想要创建一个接受 `int*` 和 `double*` 参数的构造函数,代码如下:
```c++
#include <boost/python.hpp>
class MyClass {
public:
MyClass(int x, double y) : x_(x), y_(y) {}
private:
int x_;
double y_;
};
MyClass* create_myclass(int* x, double* y) {
return new MyClass(*x, *y);
}
BOOST_PYTHON_MODULE(my_module) {
boost::python::class_<MyClass>("MyClass", boost::python::init<int, double>())
.def("__init__", boost::python::make_constructor(&create_myclass));
boost::python::def("create_myclass", &create_myclass, boost::python::return_value_policy<boost::python::manage_new_object>());
}
```
然后在 Python 中可以使用 `create_myclass` 函数来创建 `MyClass` 实例:
```python
import my_module
x = 10
y = 20.0
my_obj = my_module.create_myclass(x, y)
```
需要注意的是,使用原始指针构造函数需要确保手动管理内存,否则可能会导致内存泄漏或者悬挂指针等问题。因此,在上面的例子中,我还使用了 `boost::python::manage_new_object` 策略来确保 Python 自动管理对象的内存。
阅读全文
相关推荐
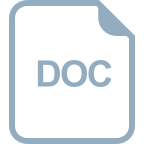
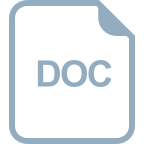
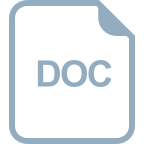
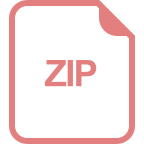
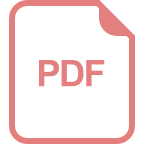
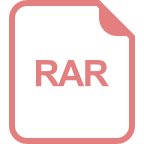
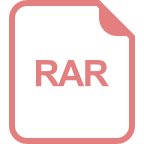
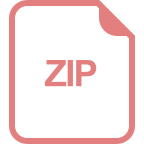
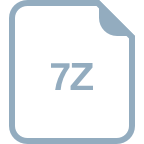
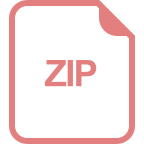
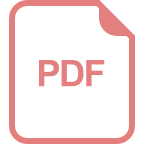
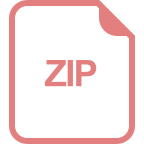
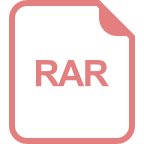
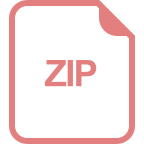
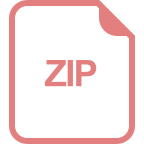
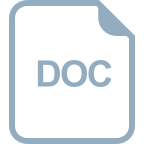
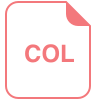
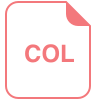
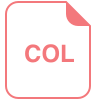