用python发送post请求
时间: 2024-10-27 20:11:54 浏览: 17
在Python中,可以使用requests库方便地发送POST请求。以下是基本步骤:
```python
import requests
# 定义URL、数据以及HTTP头部(如果有需要)
url = 'https://example.com/api/endpoint'
data = {'key1': 'value1', 'key2': 'value2'} # 请求体的数据
headers = {
'Content-Type': 'application/json', # 根据API要求设置内容类型
'Authorization': 'Bearer your_token' # 如果有认证信息
}
# 发送POST请求
response = requests.post(url, json=data, headers=headers)
# 检查响应状态码
if response.status_code == 200:
print('Post request successful')
# 获取响应数据
response_data = response.json()
# 进行后续处理
else:
print(f'Request failed with status code {response.status_code}')
相关问题
用PYTHON发送POST请求
### 回答1:
你好!下面是在 Python 中发送 POST 请求的示例代码:
```
import requests
url = 'http://www.example.com/api/v1/create'
data = {'key': 'value'}
response = requests.post(url, data=data)
print(response.status_code)
print(response.text)
```
在这段代码中,我们首先导入了 `requests` 库,然后指定了目标 URL 和需要发送的数据。接着,使用 `requests.post` 函数发送了一个 POST 请求,并将响应存储在变量 `response` 中。最后,我们输出了响应的状态码和文本内容。
注意,这段代码仅作为示例,你需要根据实际情况调整 URL 和数据内容。同时,还可能需要设置 HTTP 头信息或者使用其他的参数来定制请求。你可以参考 `requests` 库的文档来了解更多相关信息。
### 回答2:
在Python中,我们可以使用`requests`库来发送POST请求。首先,我们需要安装`requests`库。可以使用以下命令来安装:
```
pip install requests
```
安装完成后,我们可以使用以下代码来发送POST请求:
```python
import requests
url = 'https://example.com/api' # 替换为实际的API地址
data = {'name': 'John', 'age': 25} # POST请求的数据
response = requests.post(url, data=data)
if response.status_code == 200:
print('请求成功!')
print('返回的数据:', response.json())
else:
print('请求失败!')
print('错误码:', response.status_code)
print('错误信息:', response.text)
```
在以上代码中,我们首先指定了要发送POST请求的URL和请求的数据。然后,使用`requests.post()`方法发送POST请求,并将返回的响应赋值给`response`变量。
接下来,我们可以通过检查`response.status_code`属性来确定请求是否成功。如果状态码为200,表示请求成功。我们可以通过`response.json()`方法来获取返回的JSON数据。
如果请求失败,我们可以通过`response.status_code`和`response.text`来获取失败的状态码和错误信息。
以上就是使用Python发送POST请求的简单示例。如果需要更复杂的请求,例如带有请求头或认证信息的请求,可以查阅`requests`库的官方文档以获取更多信息。
### 回答3:
要用Python发送POST请求,可以使用requests库。首先,需要在Python环境中安装这个库。可以通过在命令行中运行`pip install requests`来安装。
接下来,需要引入requests库,并使用其post方法来发送POST请求。代码示例如下:
```python
import requests
url = 'http://example.com/api' # 请求的URL
data = {'username': 'user', 'password': 'pass'} # 请求的参数
response = requests.post(url, data=data) # 发送POST请求
print(response.status_code) # 打印响应状态码
print(response.text) # 打印响应内容
```
在上面的代码中,首先指定了请求的URL和参数。然后使用`requests.post`方法发送POST请求,将URL和参数作为参数传递给该方法。最后,可以通过`response.status_code`和`response.text`来获取响应的状态码和内容。
需要注意的是,如果请求的URL需要添加其他头部信息、认证信息或代理服务器等,可以在`requests.post`方法中添加相应的参数配置。详见requests库的官方文档,可以更灵活地进行POST请求的设置。
另外,要发送JSON格式的数据可以使用`requests.post`方法中的`json`参数。示例如下:
```python
import requests
import json
url = 'http://example.com/api' # 请求的URL
data = {'username': 'user', 'password': 'pass'} # 请求的JSON格式数据
response = requests.post(url, json=data) # 发送POST请求
print(response.status_code) # 打印响应状态码
print(response.text) # 打印响应内容
```
以上就是使用Python发送POST请求的基本步骤和代码示例。
用python 发送post 请求失败
发送 POST 请求可以使用 Python 的 `requests` 库。首先,你需要安装这个库:
```
pip install requests
```
然后,你可以使用以下代码发送 POST 请求:
```python
import requests
url = 'https://example.com/api/data'
data = {'key': 'value'}
response = requests.post(url, data=data)
print(response.json())
```
其中,`url` 是请求的地址,`data` 是请求的数据,`response` 是请求的响应。这个例子中,我们将数据以字典的形式传递给了 `data` 参数,并使用 `json()` 方法将响应解析为 JSON 格式。
如果你的请求需要认证,你可以使用 `auth` 参数提供用户名和密码。例如:
```python
import requests
url = 'https://example.com/api/data'
data = {'key': 'value'}
auth = ('username', 'password')
response = requests.post(url, data=data, auth=auth)
print(response.json())
```
如果你的请求需要发送 JSON 数据,你可以使用 `json` 参数代替 `data` 参数。例如:
```python
import requests
url = 'https://example.com/api/data'
data = {'key': 'value'}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, json=data, headers=headers)
print(response.json())
```
这个例子中,我们使用 `json` 参数代替 `data` 参数发送 JSON 格式的数据,并设置了请求头的 `Content-Type` 为 `application/json`。
阅读全文
相关推荐
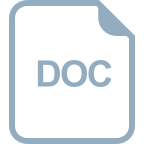
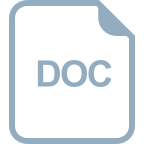
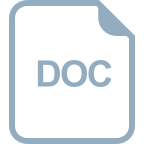












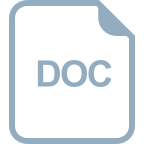
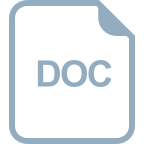