c语言实现booth算法,求补码的一位乘运算
时间: 2024-12-18 08:18:36 浏览: 18
Booth算法是一种用于计算二进制补码整数乘法的低复杂度方法,它主要用于减小乘法操作的位数,特别适合于处理器硬件实现。Booth算法的核心思想是将乘数表示成两个较小的数相加的形式,然后通过移位和减法操作来进行快速计算。
以下是使用C语言简单实现Booth算法的步骤:
```c
#include <stdio.h>
#include <inttypes.h>
// 定义Booth算法函数
void boothMultiply(int16_t multiplier, int16_t multiplicand) {
uint8_t boothArray[4];
int bitIndex = 0;
// 初始化 Booth 系列
for (int i = 0; i <= 3; ++i) {
if ((multiplier >> bitIndex) & 1) {
boothArray[i] = multiplicand << (3 - i);
}
bitIndex += 2;
}
// 计算结果
uint16_t product = 0;
for (uint8_t i = 0; i < 4; ++i) {
product |= boothArray[i]; // 右移并按位或
if (product & (1 << 15)) { // 检查最高位是否溢出
product -= 1 << 16; // 如果有溢出,左移一位并减去最大值
}
}
printf("Booth算法的结果: %d\n", product);
}
int main() {
int16_t multiplier = -7; // 负数乘数示例
int16_t multiplicand = 10; // 正数乘数示例
boothMultiply(multiplier, multiplicand);
return 0;
}
```
在这个例子中,我们首先将负数乘数转换为其两倍长的二进制形式,并初始化Booth数组。然后依次处理每个位,执行右移、按位或以及溢出检查。最后,将计算结果还原回正常的长度。
阅读全文
相关推荐
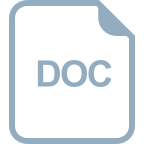
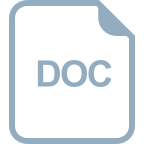
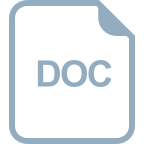


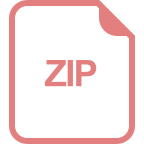
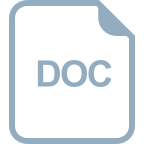
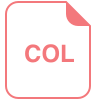
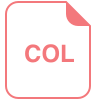


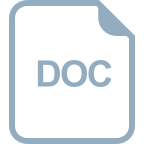
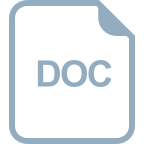
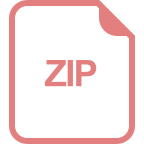
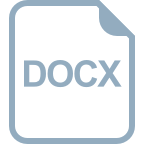