遗传算法解决选址问题代码
时间: 2023-09-11 21:06:24 浏览: 56
以下是一个简单的遗传算法代码,用于解决选址问题。该代码使用 Python 编写,需要使用 NumPy 和 Matplotlib 库。
```python
import numpy as np
import matplotlib.pyplot as plt
# 参数设置
num_locations = 50 # 地点数量
pop_size = 100 # 种群大小
num_generations = 100 # 迭代次数
mutation_rate = 0.01 # 变异率
tournament_size = 5 # 锦标赛选择大小
# 生成随机地点
locations = np.random.rand(num_locations, 2)
# 评估函数
def evaluate_fitness(population):
fitness = np.zeros(pop_size)
for i in range(pop_size):
distances = np.linalg.norm(locations - population[i], axis=1)
fitness[i] = np.sum(distances)
return fitness
# 锦标赛选择
def tournament_selection(population, fitness):
tournament = np.random.choice(pop_size, tournament_size, replace=False)
tournament_fitness = fitness[tournament]
winner = tournament[np.argmin(tournament_fitness)]
return population[winner]
# 交叉
def crossover(parent1, parent2):
child = np.zeros(2)
crossover_point = np.random.randint(2)
child[:crossover_point] = parent1[:crossover_point]
child[crossover_point:] = parent2[crossover_point:]
return child
# 变异
def mutate(child):
for i in range(2):
if np.random.rand() < mutation_rate:
child[i] = np.random.rand()
return child
# 初始化种群
population = np.random.rand(pop_size, 2)
# 迭代
for generation in range(num_generations):
fitness = evaluate_fitness(population)
fittest_individual = population[np.argmin(fitness)]
print("Generation:", generation, "Best fitness:", np.min(fitness))
new_population = np.zeros((pop_size, 2))
for i in range(pop_size):
parent1 = tournament_selection(population, fitness)
parent2 = tournament_selection(population, fitness)
child = crossover(parent1, parent2)
child = mutate(child)
new_population[i] = child
population = new_population
# 绘制结果
plt.scatter(locations[:,0], locations[:,1])
plt.plot(fittest_individual[0], fittest_individual[1], 'ro')
plt.show()
```
该代码使用随机生成的地点,通过遗传算法找到一个最优地点。每个个体(即一个地点)的适应度是到其他所有地点的距离之和。遗传算法的主要步骤包括:
1. 初始化种群
2. 对于每一代,计算每个个体的适应度
3. 选择两个个体进行交叉和变异,生成新的个体
4. 用新的个体替换旧的个体,继续迭代,直到达到指定的迭代次数
最终,算法将找到一个最优地点,并将其绘制在地图上。
相关推荐
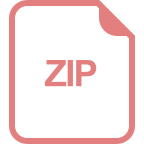














