使用golang写一个代码,使用启发式搜索A点10格范围内离B点最近的点
时间: 2023-08-31 19:36:43 浏览: 67
### 回答1:
下面是一个示例代码,它使用了 A* 搜索算法来找到离目标点 B 最近的点。在这里,我们假设 A 点和 B 点的坐标都是 (x, y) 形式的整数坐标。
```
package main
import (
"fmt"
"math"
)
type Point struct {
x, y int
}
func (p Point) Distance(target Point) float64 {
return math.Sqrt(math.Pow(float64(p.x-target.x), 2) + math.Pow(float64(p.y-target.y), 2))
}
func (p Point) NextPoints() []Point {
// 这里是扩展点的代码,它返回周围可以走到的点
return []Point{
{p.x + 1, p.y},
{p.x - 1, p.y},
{p.x, p.y + 1},
{p.x, p.y - 1},
}
}
func (p Point) String() string {
return fmt.Sprintf("(%d, %d)", p.x, p.y)
}
func astar(start, target Point) ([]Point, float64) {
// closedSet是已经走过的点,openSet是下一步要走的点
closedSet := make(map[Point]bool)
openSet := map[Point]float64{start: start.Distance(target)}
// parents用来记录每个点的父结点,用于最后打印路径
parents := make(map[Point]Point)
for len(openSet) > 0 {
// 找到f值最小的点
current := Point{}
f := math.MaxFloat64
for point, pointF := range openSet {
if pointF < f {
current = point
f = pointF
}
}
// 如果当前点是目标点,打印路径
if current == target {
path := []Point{}
for current != start {
path = append([]Point{current}, path...)
current = parents[current]
}
return append([]Point{start}, path...), f
}
// 从openSet中删除,加入closedSet
delete(openSet, current)
closedSet[current] =
### 回答2:
使用Golang编写一个代码实现A点10格范围内离B点最近的点的启发式搜索。
首先,我们需要定义两个点的结构体:Point和Distance。
```go
type Point struct {
X, Y int
}
type Distance struct {
Point Point
D int
}
```
然后,我们可以编写一个函数来计算两个点之间的欧几里得距离。
```go
func calcDistance(p1, p2 Point) int {
return (p1.X-p2.X)*(p1.X-p2.X) + (p1.Y-p2.Y)*(p1.Y-p2.Y)
}
```
接下来,我们可以编写一个函数来实现启发式搜索,找到A点10格范围内离B点最近的点。
```go
func findNearestPoint(A, B Point) Point {
minDistance := math.MaxInt32
nearestPoint := Point{}
for i := A.X - 10; i <= A.X+10; i++ {
for j := A.Y - 10; j <= A.Y+10; j++ {
if i >= 0 && i < mapWidth && j >= 0 && j < mapHeight {
currentPoint := Point{i, j}
currentDistance := calcDistance(currentPoint, B)
if currentDistance < minDistance {
minDistance = currentDistance
nearestPoint = currentPoint
}
}
}
}
return nearestPoint
}
```
在这个函数中,我们遍历A点10格范围内的所有点,计算每个点到B点的距离,并更新最近距离和最近点的值。
最后,我们可以在主函数中调用这个函数,并打印出结果。
```go
func main() {
A := Point{1, 1}
B := Point{5, 5}
nearestPoint := findNearestPoint(A, B)
fmt.Println(nearestPoint)
}
```
这个代码将会打印出在A点10格范围内离B点最近的点的坐标。
请注意,上述代码只是一个简单的示例,具体实现可能需要根据实际需求进行调整和优化。
### 回答3:
使用golang编写一个代码,通过启发式搜索算法找出在A点10格范围内离B点最近的点。
首先,我们需要定义一个表示点的结构体,包含点的坐标x和y。代码如下:
type Point struct {
x, y int
}
接下来,我们需要实现一个函数来计算两点之间的欧氏距离。代码如下:
func Distance(p1, p2 Point) float64 {
dx := p1.x - p2.x
dy := p1.y - p2.y
return math.Sqrt(float64(dx*dx + dy*dy))
}
接下来,我们可以编写启发式搜索函数,通过遍历A点10格范围内的所有点,计算每个点到B点的距离并保存最小距离的点。代码如下:
func FindNearestPoint(A, B Point) Point {
minDist := math.Inf(1)
var nearestPoint Point
for i := A.x - 10; i <= A.x+10; i++ {
for j := A.y - 10; j <= A.y+10; j++ {
currentPoint := Point{i, j}
dist := Distance(currentPoint, B)
if dist < minDist {
minDist = dist
nearestPoint = currentPoint
}
}
}
return nearestPoint
}
最后,我们可以在main函数中调用FindNearestPoint函数,并输出结果。代码如下:
func main() {
A := Point{2, 3}
B := Point{5, 7}
nearestPoint := FindNearestPoint(A, B)
fmt.Println("离B点最近的点是:", nearestPoint)
}
以上代码使用启发式搜索算法,遍历A点10格范围内的所有点,计算每个点到B点的距离,然后返回最小距离的点。输出结果为离B点最近的点的坐标。
相关推荐
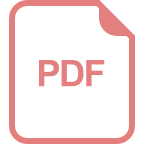














